Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial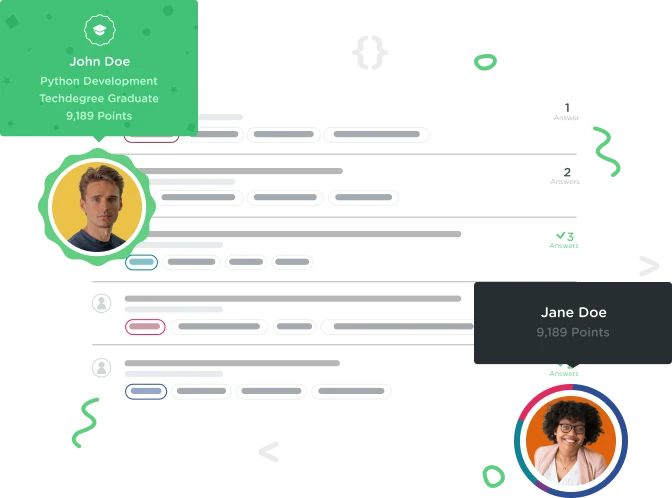

pooja goyal
245 PointsThe following table describes my room temperature preferences. Print the message from the table when a user enters a num
The following table describes my room temperature preferences. Print the message from the table when a user enters a number in the corresponding range. For example, if temperature is 21 the code should print "Just right." to the screen.
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature<21)
{
console.writeline("too cold!");
}
elseif(temperature>=21 && temperature<=22)
{
console.writeline("just right.");
}
else
{
console.writeline("too hot!");
}
4 Answers
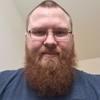
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsThere are two problems here:
- else if statements are written like this else if() and not like this elseif()
- C# is a case-sensitive language and therefor console.writeline() should be Console.WriteLine()
The code should look something like this:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature<21)
{
Console.WriteLine("too cold!"); // notice the Console.WriteLine instead of console.writeline
}
else if(temperature>=21 && temperature<=22) // here is a space between else and if
{
Console.WriteLine("just right.");
}
else
{
Console.WriteLine("too hot!");
}
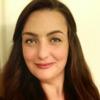
Jennifer Nordell
Treehouse TeacherHi there! Your logic is spot on, but your syntax is a bit off. The first error is in the elseif
. In C# we write it as two separate words else if
. Your other syntax errors are about capitalization.
In each case, you have written console.writeline
, but the capitalization must be Console.WriteLine
. This is how C# has chosen to implement the System.Console
class and the method name is WriteLine
. Without this capitalization it is looking for variables which were never defined.
Hope this helps!
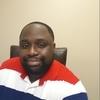
Christian Tisby
9,329 PointsI don't remember learning anything about the && for adding an additional int in the videos up to this point, maybe that's why I couldn't figure this out.

tipi99
3,019 PointsSince temperature is an Int you can also write: else if (temperature == 21 || temperature == 22)

Brandon lile
1,271 PointsWhat did I do wrong?
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature <21)
{
Console.WriteLine("Too cold!");
}
else if (temperature ==21 && temperature <=22)
{
Console.WriteLine("Just right.");
}
else()
{
Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that the code renders properly in the forums.
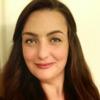
Jennifer Nordell
Treehouse TeacherThis should have been submitted as a separate question. You have a syntax error and a logic flaw. The parentheses after the else
is causing the syntax error. An else
statement takes no arguments.
The logic flaw is in your else if
. You say if the temperature is 21 and the temperature is less than or equal to 22 then it's just right. This means that it cannot be 22. Both conditions must be true for this to evaluate to true. While 22 is less than or equal to 22, it is not equal to 21, so it will fail. The condition should be:
else if (temperature >= 21 && temperature <= 22)
Now if the temperature is 21, 22 or anywhere in between it will pass. Hope this helps!