Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial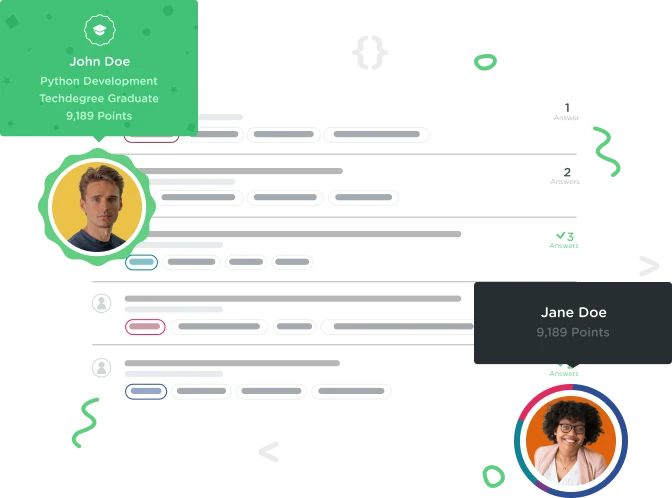

PoJung Chen
5,856 PointsThe writing sequence of schema creation in mongoose
I am creating Schema and instance method for the Schema in Mongoose. However, I faced some problems.
This is the part of code in models
const AnswerSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
updatedAt: {type: Date, default: Date.now},
votes: {type: Number, default: 0}
})
AnswerSchema.method('update', function (updates, callback) {
Object.assign(this, updates, {updatedAt: new Date()})
this.parent().save(callback)
})
AnswerSchema.method('vote', function (vote, callback) {
if (vote === 'up') {
this.votes += 1
} else {
this.votes -= 1
}
this.parent().save(callback)
})
const QuestionSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
answers: [AnswerSchema]
})
and it will show me that The #update method is not available on EmbeddedDocuments when I get the PUT request in my routes.
router.put('/:qID/answers/:aID', (req, res, next) => {
req.answer.update(req.body, function (err, result) {
if (err) return next(err)
res.json(result)
})
})
However, if I changed the sequence of my code, it works fine:
const AnswerSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
updatedAt: {type: Date, default: Date.now},
votes: {type: Number, default: 0}
})
AnswerSchema.method('update', function (updates, callback) {
Object.assign(this, updates, {updatedAt: new Date()})
this.parent().save(callback)
})
AnswerSchema.method('vote', function (vote, callback) {
if (vote === 'up') {
this.votes += 1
} else {
this.votes -= 1
}
this.parent().save(callback)
})
const QuestionSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
answers: [AnswerSchema]
})
Could someone explain why this happens for me??
Zack Lee
Courses Plus Student 17,662 PointsZack Lee
Courses Plus Student 17,662 PointsI don't see any difference between the 2 code blocks you posted. Also theres a lack of semi colons in pretty much every place they're needed? if this reflects your actual code, there could be unexpected behavior because of that.