Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial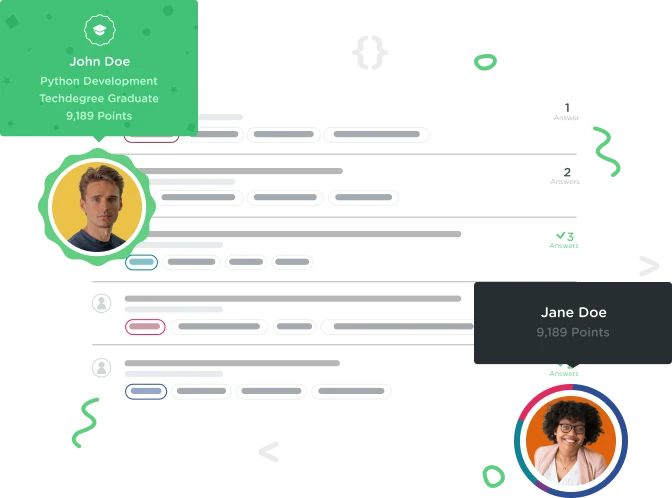

Hunter Shaw
2,187 PointsThere was an error with your code: ReferenceError: Can't find variable: num1
I can't seem to figure this out. Could anyone help me understand this more, and maybe fix my broken code?
Thanks!
// There was an error with your code: ReferenceError: Can't find variable: num1
function max(num1, num2) {
var num1;
var num2;
return num1, num2;
}
max(5, 7);
if (num1 > num2) {
document.write(num1);
} else {
document.write(num2);
}
2 Answers
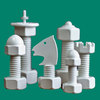
Steven Parker
231,084 PointsHere's a few hints and suggestions:
- do not declare passed-in arguments as variables inside the function
- you can only return one of the values
- you need a comparison test to determine which value to return
- for task 2 you will only need one more line of code after the function
- you won't need to use "document.write" (you will use "alert" instead)

Hunter Shaw
2,187 Pointsfunction max(num1, num2) {
var numbers = num1;
numbers += num2;
if (num1 > num2) {
return num1;
} else {
return num2;
}
}
max(5, 7);
/*
1. do not declare passed-in arguments as variables inside the function
2. you can only return one of the values
3. you need a comparison test to determine which value to return
4. for task 2 you will only need one more line of code after the function
5. you won't need to use "document.write" (you will use "alert" instead)
*/
Thank you so much, the suggestions helped a lot. I just didn't understand that you could have a return in the middle of the code block. I though that returns are ALWAYS on the bottom, but in this case that isn't turn. Return will end the program and the if statement is what was needed. Thanks so much! A little confusing at times but I think with some practice I will be-able to code better in no time! :)
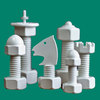
Steven Parker
231,084 PointsNote that you also don't need either of the lines with "numbers". It gets assigned but never used.
Hunter Shaw
2,187 PointsHunter Shaw
2,187 PointsThe coding challenge is to get the larger number of the two. Doesn't this work?