Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial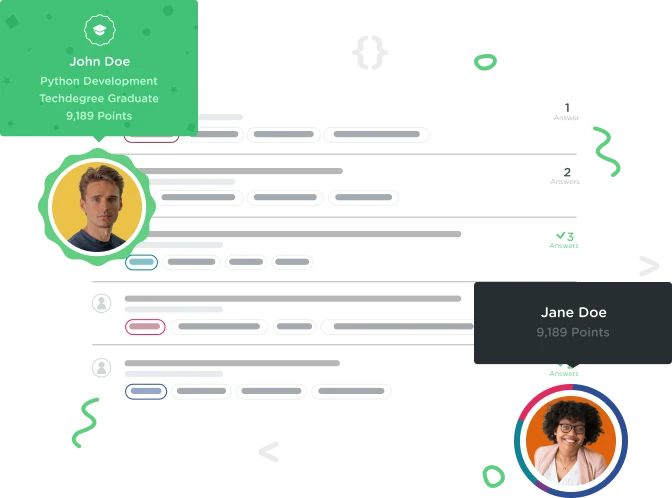
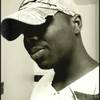
Lee Reynolds Jr.
5,160 PointsThrowing an Exception
I can't seem to figure out what is going wrong with my code. It's passing through the checker without and errors but I still can't get it to pass the exercise.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if((laps--) < 0){
System.out.println("There isn't enough power for another lap.");
}
try{
mBarsCount -= laps;
}catch(IllegalArgumentException iae){
System.out.println("Not enough battery remains.");
System.out.printf("The error was: %s\n", iae.getMessage());
}
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer
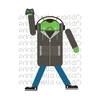
Stephen Bone
12,359 PointsHi Lee
If you think about it in two parts - This task is the first part where you set up to test for an IllegalArgumentException. Then in the next part use try to run the code and catch the exception from the test should it trigger. Basically you are doing part two without doing part one, if that makes sense.
So the way Craig works through it in the video is to create a new variable to hold the possible new value. Then uses an if statement to test whether it would do something we don't want it to. If it does then we create a new IllegalArgumentException along with the message in the task otherwise mBarsCount should be updated.
This should then pass this task which leads to the next task where you need to catch this exception - similar to the code you have here.
I've included my code below as a guide.
Hope it helps!
Stephen
public void drive(int laps) {
// Other driving code omitted for clarity purposes
int newBarsCount = mBarsCount - laps;
if (newBarsCount < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount = newBarsCount;
}
Lee Reynolds Jr.
5,160 PointsLee Reynolds Jr.
5,160 PointsThanks a bunch. That worked like a charm.