Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial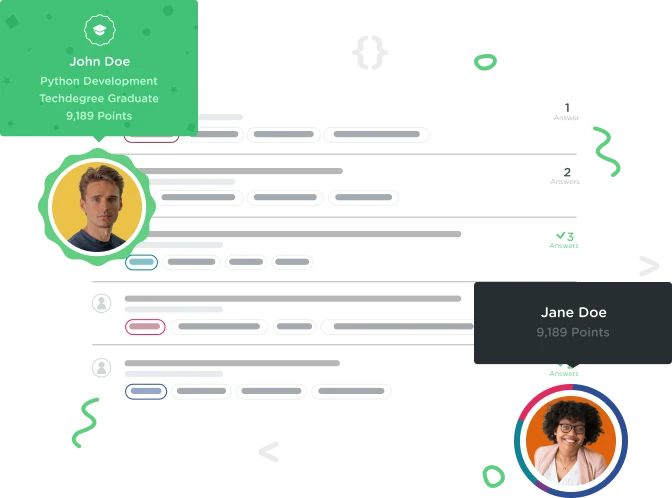
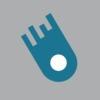
Gabriel E
8,916 PointsTotally and cluelessly stuck on challenge
Hi there,
I'm working on the 1st task of the 4-part challenge. I'm not sure what to do on this. I have tried just about everything I can think of that I have learned. Any help or helpful code would be appreciated. Thanks!
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
3 Answers
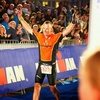
Steve Hunter
57,712 PointsHi Gabriel,
The first bit needs you to add a construtor to the Forum class. That looks like:
public Forum (String topic) {
mTopic = topic;
}
Next, you need to add the relevant private fields based on the existing constructor. It has two parameters called firstName
and lastName
, so you just need to create member variables with appropriate names as well as getter methods:
public class User {
private String mFirstName;
private String mLastName;
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName; // set the member variables here too
mLastName = lastName;
}
}
There's quite a bit to add in that last one!! And the next part is a large one too - so I'll post this now and add the remaining bits in a minute when I've worked through it.
Steve.
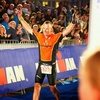
Steve Hunter
57,712 Points... continued ...
The next part wants tou to add a constructor to the ForumPost
class - it gives you the parameters and you can see the member variables. You also need to add a getter for mDescription
.
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription() {
return mDescription;
}
Now, if I remember rightly, comes the tricky bit!! First you need to uncomment the addPost
method in Forum.java
. Also, we need to uncomment the lines inside Example.java
- especially the last one as that will drive the errors that you need to fix. You follow your nose through the errors and fix them one by one. Let's have a go!
The first line in main
is:
Forum forum = new Forum("Java");
Will that work? Yes it will - the constructor in the Forum
class takes a string. So that's fine ... move on ...
The next line is:
User author = new User();
We wrote the constructor for this - it needs a first and last name. The comment above it says: // Take the first two elements passed args
so now we know where to get the parameters from!
User author = new User(args[0], args[1]);
Next up is a new ForumPost
. Again, we wrote its constructor so know what it wants. Just where to find those things?! It takes an author, title and description. We have created one of those above this line - the others we're invited to add something personal. I declined:
ForumPost post = new ForumPost(author, "Title", "Description");
I know that's a lot of typing and reading - feel free to ask questions!
I do hope it helps.
Steve.
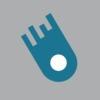
Gabriel E
8,916 PointsHi Steve,
Thanks so much, I passed the challenge! One thing I don't quite understand. The line you wrote that I am supposed to uncomment, User author = new User();, I ended up not needing that because it was already defined, so once I commented that, I was able to complete the tasks. Just wondering why this was? Thanks!
Gabriel
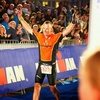
Steve Hunter
57,712 PointsYou may have added both the original line,
User author = new User();
and the modified one that added the args[]
parameters?
User author = new User(args[0], args[1]);
My walk-through was just that - it builds each line as required, step-by-step.
Glad you got it sorted!
Steve.