Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial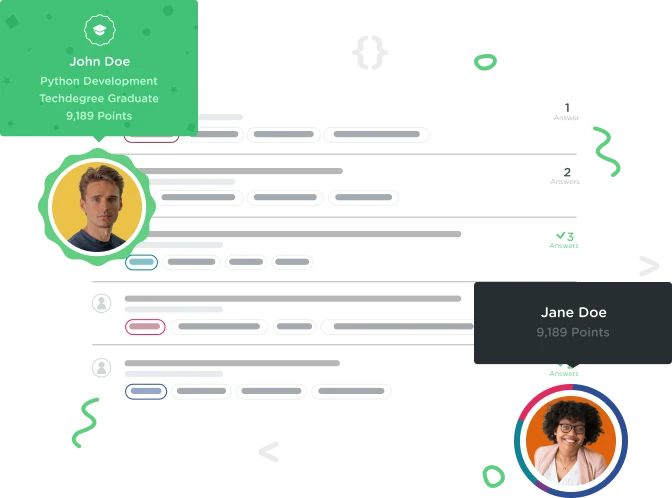

jerome moore
5,005 PointsTreeHouse Blog post on creating Jquery Ajax PHP mail form 500 error
I was following the blog post from http://blog.teamtreehouse.com/create-ajax-contact-form and I got all steps to work on my localhost/MAMP config EXCEPT getting the php script to actually mail the file contents. It keeps giving me a 500 error. did not know how to debug so If I can get some helpful tips, that would be awesome. PHP version on MAMP is 7.0.0
EDIT sorry - forgot something :-/
php code first:
<?php
// Only process POST reqeusts.
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Get the form fields and remove whitespace.
$name = strip_tags(trim($_POST["name"]));
$name = str_replace(array("\r","\n"),array(" "," "),$name);
$email = filter_var(trim($_POST["email"]), FILTER_SANITIZE_EMAIL);
$message = trim($_POST["message"]);
// Check that data was sent to the mailer.
if ( empty($name) OR empty($message) OR !filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Set a 400 (bad request) response code and exit.
http_response_code(400);
echo "Oops! There was a problem with your submission. Please complete the form and try again.";
exit;
}
// Set the recipient email address.
// FIXME: Update this to your desired email address.
$recipient = "jeromemoore@usa.com";
// Set the email subject.
$subject = "New contact from $name";
// Build the email content.
$email_content = "Name: $name\n";
$email_content .= "Email: $email\n\n";
$email_content .= "Message:\n$message\n";
// Build the email headers.
$email_headers = "From: $name <$email>";
// Send the email.
if (mail($recipient, $subject, $email_content, $email_headers)) {
// Set a 200 (okay) response code.
http_response_code(200);
echo "Thank You! Your message has been sent.";
} else {
// Set a 500 (internal server error) response code.
http_response_code(500);
echo "Oops! Something went wrong and we couldn't send your message.";
}
} else {
// Not a POST request, set a 403 (forbidden) response code.
http_response_code(403);
echo "There was a problem with your submission, please try again.";
}
?>
The Javascript:
<script>
$(document).ready(function($) {
var form = $('#ajax-contact');
$(form).submit(function(event) {
var formData = {
'name' : $('#name').val(),
'email' : $('#email').val(),
'message' : $('#message').val()
};
//var formData = $(form).serialize();
event.preventDefault();
console.log(formData);
$.ajax({
type: "POST",
url: $(form).attr('action'),
data: formData,
success: function(msg){
alert("form post success!");
//$("#stayInformed").modal('hide');
},
error: function(){
alert("form post failed");
}
});
});
});
</script>
The Form:
<div class="footer-right">
<p class="holla">Contact Us</p>
<form method="post" id="ajax-contact" name="emailform" action="email.php">
<input type="text" name="name" placeholder="Name" id="name" required>
<input type="text" name="email" placeholder="Email" id="email" required>
<textarea name="message" placeholder="Message" id="message" required></textarea>
<button type="submit" name='submit' value="submit">Send</button>
</form>
</div>
Any Ideas?
2 Answers

jerome moore
5,005 PointsAny Ideas Ashley?
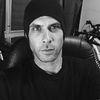
jack AM
2,618 PointsHi Jerome,
What mail server are you using? Before I delve into anything else (because your code looks fine), I don't want to assume that you're not using one, but I happened to notice that in the tutorial you linked, the author assumes that those following has one up and running already. I assume this, because he doesn't mention it anywhere in the tutorial, however one is needed to send email using php's mail() function, and would be a good culprit as to why you're receiving that 500 error.

jerome moore
5,005 PointsHello Jack,
Thanks for the insight. I am using sendmail on a linux ubuntu 14.04 box. It gives a success message, but does not send mail. not sure what the issue is, but I suspect it is a configuration issue with sendmail?
Codin - Codesmite
8,600 PointsCodin - Codesmite
8,600 PointsHi Jerome, can you post your code here so that we check and see what may be going wrong.