Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial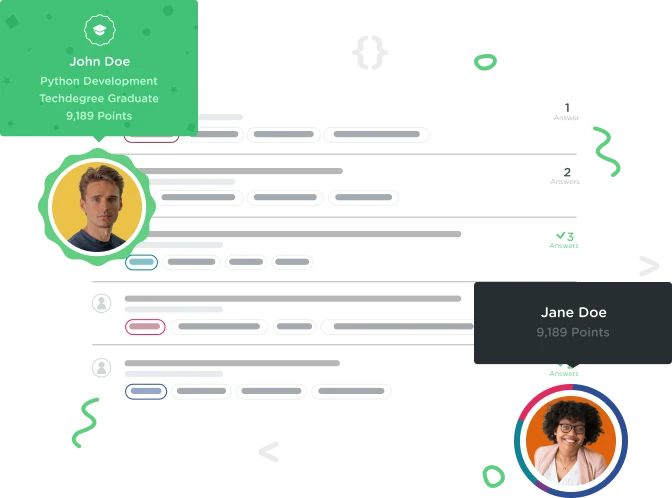

Oscar Bale
3,416 PointsTreehouseDefense program error CS1729
I had just finished coding the Level.cs part of the program when I noticed this error:
"Game.cs(9,27): error CS1729: The type TreehouseDefense.Tower' does not contain a constructor that takes
0' arguments"
Game.cs code: class Game { public static void Main() { Tower tower = new Tower(); Map map = new Map(8, 5);
try
{
Path path = new Path(
new [] {
new MapLocation(0, 2, map),
new MapLocation(1, 2, map),
new MapLocation(2, 2, map),
new MapLocation(3, 2, map),
new MapLocation(4, 2, map),
new MapLocation(5, 2, map),
new MapLocation(6, 2, map),
new MapLocation(7, 2, map),
}
);
}
catch(OutOfBoundsException ex)
{
Console.WriteLine(ex.Message);
}
catch(TreehouseDefenseException)
{
Console.WriteLine("Unhandled TreeHouseDefenseException");
}
catch(Exception ex)
{
Console.WriteLine("Unhandled Exception" + ex);
}
}
Map.cs code: class Map { public readonly int Width; public readonly int Height;
public Map(int width, int height)
{
Width = width;
Height = height;
}
public bool OnMap(Point point)
{
return point.X >= 0 && point.X < Width && point.Y >= 0 && point.Y < Height;
}
}
MapLocation.cs code: class MapLocation : Point { public MapLocation(int x, int y, Map map) : base(x, y) { if (!map.OnMap(this)) { throw new OutOfBoundsException(x + "," + y + " is outside the boundaries of the map"); } }
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location) <= range;
}
}
I am unsure of how to fix this,
Any help would be appreciated.
1 Answer
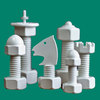
Steven Parker
231,269 PointsI'd need to see the code in the "Tower" module to be sure, but the error implies that the Tower constructor requires one or more arguments, but on line 9 the code is attempting to create an instance without any ("new Tower()
").

Oscar Bale
3,416 Points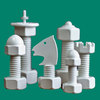
Steven Parker
231,269 PointsIt's what I guessed, In Game.cs on line 9 the code is attempting to create a new "Tower" instance without passing any argument ("new Tower()").
But in Tower.cs line 11 the constructor is defined to require a MapLocation argument. You'd need to pass one to create the Tower instance.
You didn't give a link to the course page you are using, but perhaps the project is being changed from using no argument to needing one and you are only partway through.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou forgot to include the Tower.cs code.
To share the entire project, make a snapshot of your workspace and post the link to it here.