Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial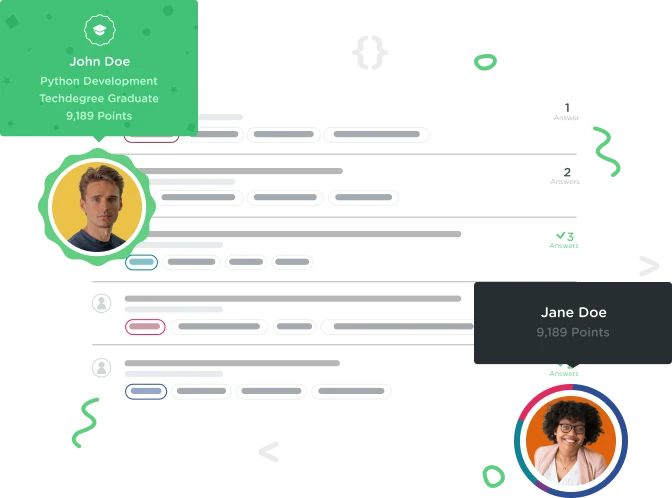

jon willey
845 Points"try" and "catch"
Can't get this to run. Don't know what I did
using System;
namespace Treehouse.FitnessFrog//namespace { class Program//class { static void Main()//method {
double runningTotal = 0;
bool keepGoing = true;
while(keepGoing)
{
//prompt user for minutes exercisesd
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
keepGoing = false;
}
else
{
try
{
double minutes = double.Parse(entry);
if(minutes <= 0)
{
Console.WriteLine(minutes + " is not an accepatble value.");
continue;
}
else if(minutes <= 10)
{
Console.WriteLine("Better than nothing");
}
else if(minutes<=30)
{
Console.WriteLine("Way to go");
}
else if(minutes <=60)
{
Console.WriteLine("Pizza tonight!");
}
else
{
Console.WriteLine("you are just showing off!");
}
}
runningTotal = runningTotal + minutes;
{
catch(FormatException)
{
console.WriteLine("That is not valid input.");
continue;
}
//add minutes exd to total
Console.WriteLine("You entered " + runningTotal + " minutes");
//Display total minutes exercised to the screen
//repeat until user quits
}
}
}
}
}
2 Answers
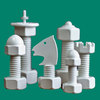
Steven Parker
241,809 PointsI'm seeing a few issues here:
- the catch block should directly follow the try block
- the catch block should be at the same level as the try block (not inside another block)
- C# is case sensitive, so console.WriteLine needs to be Console.WriteLine
- you were missing some ending braces (or had stray open braces)
-
acceptable was misspelled ("accepatble")
Here's the code with those issues repaired:
using System;
namespace Treehouse.FitnessFrog //namespace
{
class Program //class
{
static void Main() //method
{
double runningTotal = 0;
bool keepGoing = true;
while(keepGoing)
{
//prompt user for minutes exercisesd
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
keepGoing = false;
}
else
{
try
{
double minutes = double.Parse(entry);
if(minutes <= 0)
{
Console.WriteLine(minutes + " is not an acceptable value.");
continue;
}
else if(minutes <= 10)
{
Console.WriteLine("Better than nothing");
}
else if(minutes <= 30)
{
Console.WriteLine("Way to go");
}
else if(minutes <= 60)
{
Console.WriteLine("Pizza tonight!");
}
else
{
Console.WriteLine("you are just showing off!");
}
//add minutes exd to total
runningTotal = runningTotal + minutes;
}
catch(FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
//Display total minutes exercised to the screen
Console.WriteLine("You entered " + runningTotal + " minutes");
}
//repeat until user quits
}
}
}
}
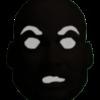
Geovanie Alvarez
21,500 PointsTry this to see if work in the catch part add a name of the variable FormatException
catch(FormatException e) // <------- here
{
console.WriteLine("That is not valid input.");
continue;
}
jon willey
845 Pointsjon willey
845 PointsThanks Steven!! Debugging is difficult:)