Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial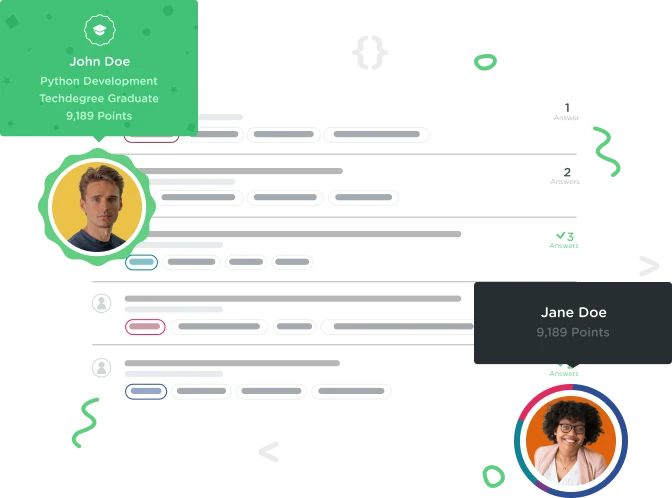

Joel Muro
2,884 Pointstry code and catch exceptions
Fix the code so that it compiles, but don't change the intent of the code.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
input = Console.ReadLine();
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}
i have no idea where to start?
2 Answers
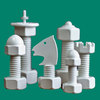
Steven Parker
231,096 PointsAssuming you'd like hints, and not just the answer:
- This challenge is about declaration and scope.
- Look carefully at where (and if!) the variables are declared
- Consider the error messages generated from the compiler.
- Each variable should have one declaration.
- If you see a variable being declared more than once, it probably needs to be declared once in a wider scope.
Another hint: there is more than one thing that must be fixed for this code to compile.

Joel Muro
2,884 PointsI see that there are 2 string output = but to different text. is that going in the right direction?
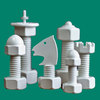
Steven Parker
231,096 PointsGood catch. This is a case where you want to move the declaration to a wider scope and convert the declarations/assignments into simple assignments. So instead of:
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
}
You might have:
string output = ""; // <-- empty assignment to appease the compiler
if (input == "quit")
{
output = "Goodbye."; // <-- just a plain assignment
}
else
{
output = "You entered " + input + "."; // <-- also just a plain assignment
}
...or perhaps eliminate the else entirely (this is a bit more than just fixing it to compile):
string output = "You entered " + input + ".";
if (input == "quit")
{
output = "Goodbye.";
}