Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial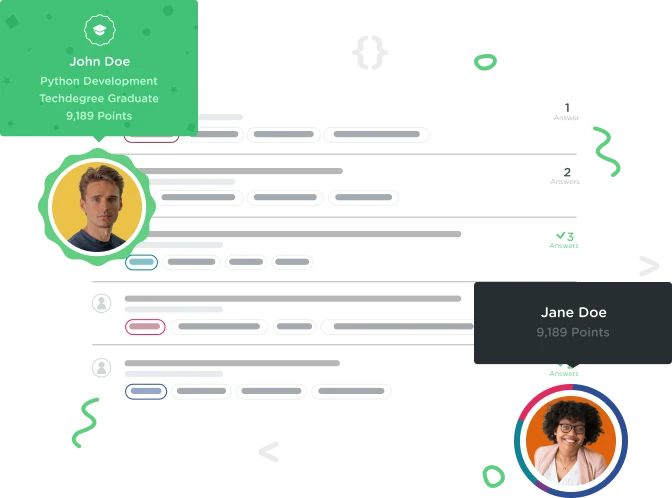
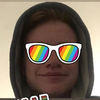
Nils Garland
18,416 PointsTrying to insert form data in to my database, please help!
Hi, I'm trying to insert form data in to my database to make a registration form. Note that I'm not bothered with saftey for now since I'm just testing this stuff out. Also I have already made the tables and stuff and I have dummy accounts that I can log in to. Please help.
Code:
$uname = $_POST['uname'];
$pass = $_POST['pass'];
$email = $_POST['mail'];
include("db.php");
$mysql = "INSERT INTO Users (username, password, email)
VALUES ($uname, $pass, $email)";
2 Answers
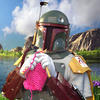
Lindsay Sauer
12,029 Points$mysql is just the query you want to run; in order to execute the query, you have to use mysql_query. This should work:
$uname = $_POST['uname'];
$pass = $_POST['pass'];
$email = $_POST['mail'];
include("db.php");
$mysql = "INSERT INTO Users (username, password, email) VALUES ($uname, $pass, $email)";
$result = mysql_query($mysql);
if (!$result) {
die('Invalid query: ' . mysql_error());
}
I know you mentioned safety, but I'd highly recommend not using MySQL and looking at MySQLi or PDO. The mysql functions were deprecated as of PHP 5.5 and were removed in PHP 7.

Maximillian Fox
Courses Plus Student 9,236 PointsUsing mysqli
<?php
// Assume we have an established connection to the database in a variable called $conn
// Make the query
$insertUserDetails = "INSERT INTO Users (username, password, email) VALUES ('$uname', '$pass', '$email')";
// Querying the table
$result = $conn->query($insertUserDetails);
// You can report the mysqli error here but if there is a problem I just show the query so I can check it, out of personal preference.
if(!$result){
die("The query was not valid. $insertUserDetails");
}
?>
In order to make this more secure, you also need to make sure you are sanitizing your user's inputs before you hit the database part. Otherwise you could open yourself up to a SQL injection attack. If you want to find out about some examples, the PHP docs has a whole section dedicated to this, with some examples that actually happened.
Another way to protect yourself from injection is to use prepared statements. I encourage you to look this up in the PHP documentation so you can explore how this works. If you find the mysqli->prepare() too limiting (sometimes you may want to set up your own abstraction layer classes with functions to query and bind a variable number of parameters for different queries, which mysqli is not so easily capable of doing) then I would look into PHP Data Objects (PDO) for this. Treehouse does a course on this too.
Nils Garland
18,416 PointsNils Garland
18,416 PointsThanks a lot Lindsay! I am using mysqli in most parts of my code bu I'm not sure what the difference is, I will have a look at it.
Thanks again!