Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial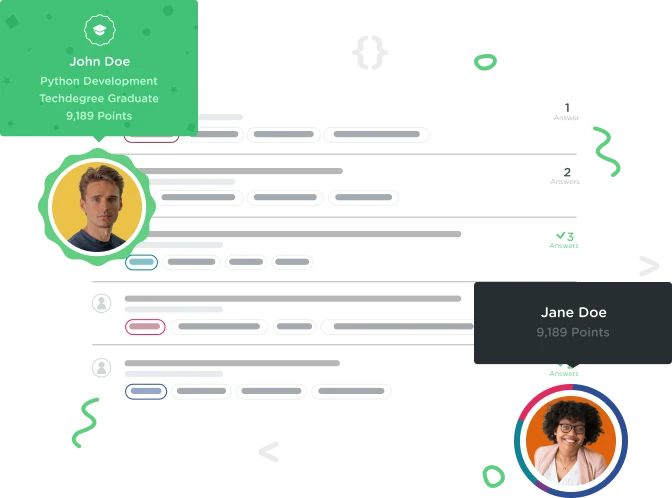

Noelle Acheson
610 PointsTrying to use isUpperCase
I'm trying to test for second character being uppercase - I get an error on the method, not sure why?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
char firstChar = fieldName.charAt(0);
char secondChar = fieldName.charAt(1);
boolean secondTest = isUpperCase(secondChar);
if (firstChar != 'm' || secondTest) {
throw illegalArgumentException("The name does not match format");
}
return fieldName;
}
}
3 Answers

Sascha Bratton
3,671 PointsLooks like you've got a few problems here.
First, isUpperCase
is a method of the Character class. So you need to call it like so:
boolean secondTest = Character.isUpperCase(secondChar);
Second, classes are case-sensitive, so illegalArgumentException
needs to be IllegalArgumentException
and also, you need to instantiate a new instance with new
like so:
throw new IllegalArgumentException("The name does not match format");
And lastly, while that will take care of your compiler errors, the return on secondTest
is going to be true
if the second character of the fieldName
parameter is, in fact, uppercase.
So in order to throw the exception if the second character is not uppercase, you need to make your condition to be if it is false, like so:
if (firstChar != 'm' || !secondTest) {
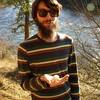
Nico Julian
23,657 PointsHi,
Since char is considered a primitive data type, it doesn't have methods attached to it. For this reason, there's a box type class, Character, which does contain methods. When you call isUpperCase(), try it like this:
boolean secondTest = Character.isUpperCase(secondChar);
if (firstChar != 'm' || !secondTest) {
throw new IllegalArgumentException("The name does not match format");
}
I added an '!' before second test since we want to throw the exception if the first character isn't 'm', or the second test isn't uppercase.

Noelle Acheson
610 PointsThanks!! I get it now!!!