Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial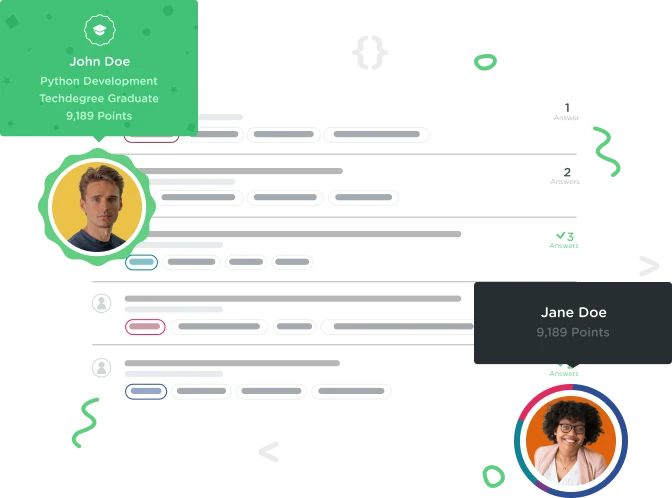

Vijesh Arora
Front End Web Development Techdegree Student 34 PointsType casting index of array?
Kindly first check this code
<?php
function indexOfArray(array $arr = [], $index = null){
if(! is_int($index)){
die("Argument is not an integer");
}
return array_key_exists($index, $arr) ? $arr[$index] : null;
}
$numbers = [
0 => 'Zero',
1 => 'One',
2 => 'Two',
3 => 'Three'
];
echo indexOfArray($numbers, 3);
// Output - Three
Now my question is, what is the purpose of writing "array" $arr... , if I remove the word array, output comes same as it is with array.
Moderator edited: markdown was added to the question to properly render the code. Please see the Markdown Cheatsheet link at the bottom of the "Add an Answer" section for tips on how to post code to the Community.
1 Answer
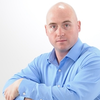
Benjamin Payne
8,142 PointsHey Vijesh,
Ben again. The purpose of type hinting an array is so that you cannot accidentally pass a different type of scalar to this function. Most IDE's will warn you if you do but the real power comes when writing Unit Tests. Unit tests will catch the bug in your code prior to deploying it. If you remove the type hint, PHP will try its hardest to continue to run by attempting to cast what you passed to it to as an array, however there is no guarantee this will work. If you didn't catch the bug and a different type was passed to the indexOfArray function then in, PHP 7 at least, you will get a TypeError.
Think of type hinting as a way to safeguard your application from bugs. In statically / strongly typed languages like Java every parameters type must be declared, as well as method and function return types.
Hopefully that helps.
Thanks,
Ben
Vijesh Arora
Front End Web Development Techdegree Student 34 PointsVijesh Arora
Front End Web Development Techdegree Student 34 PointsThanks, easy to understood. I am very new in such terms, so might be getting difficult to understand.