Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial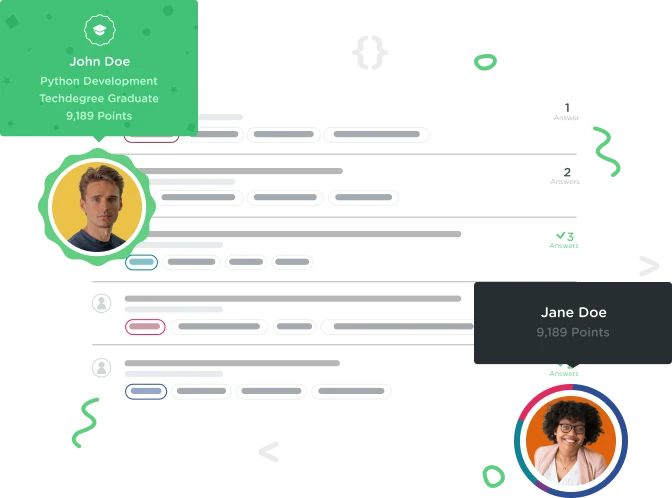

Tony Brackins
28,766 PointsUnderscore
Still confused about what the underscore does in this video
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
}
1 Answer
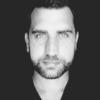
Jhoan Arango
14,575 PointsHello Tony:
When creating a function, you can give it parameters, and these parameters can be named. They use what's called a Local Parameter Name and an External Parameter Name. The Local Parameter Name is used within the definition of the function, and the External Parameter Name is used when calling the function and passing it arguments.
As a default behavior, the first parameter always omits it's External Parameter Name, but the subsequent parameters use their Local Parameter Name as their External Parameter Name.
All of this, is in Swift 2.0 and above. On Swift 1.2 there were different rules. But the underscore still the same.
For example:
// Let's create a function
func add(number: Int, otherNumber: Int) -> Int {
let sum: Int = number + otherNumber
return sum
}
// When calling this function, it looks like this
add(2, otherNumber: 8) // It will return 10
// It does not make much sense to say "add 2 other number"
// It does not sound clean right ?
We can fix that and make it better with a better external parameter name.
// Same function, but now we are giving it a different External Parameter Name
// by putting the name before the local parameter name.
func add(number: Int,plus otherNumber: Int) -> Int {
// Here we are using the local parameter name for the
// function definition.
let sum: Int = number + otherNumber
return sum
}
// Now when we call the function it looks like this:
add(2, plus: 8)
// Now it sounds much better .. "add 2 plus 8"
Now, if you do not want to use the external parameter name, you use the underscore.
func add(number: Int, _ otherNumber: Int) -> Int {
let sum: Int = number + otherNumber
return sum
}
// When we call the function, you do not have to use
// the external parameter name since we are ignoring it
// with the underscore.
add(2,8) // It will return 10
I hope this makes a lot more sense to you.
If this answer helped you, remember to help others by selecting it as best answer.
Good Luck.
Tony Brackins
28,766 PointsTony Brackins
28,766 PointsMost definitely helped. Thx!!