Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial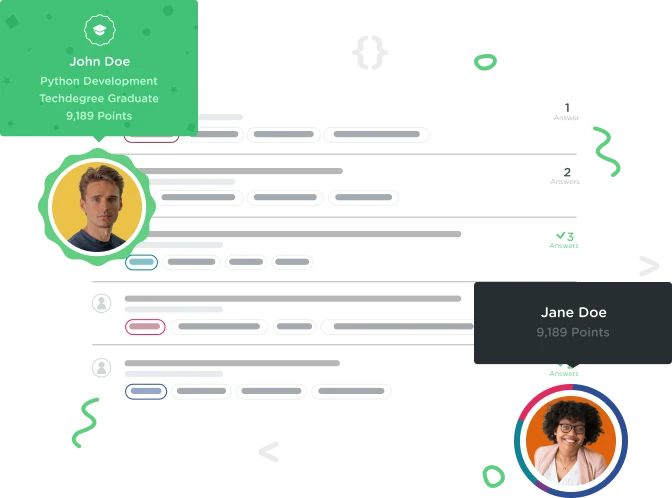
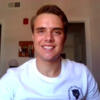
Evan Welch
1,815 PointsUnderstanding the difference between static methods and instance methods in C#
How is the DateTime.AddDays method not a static method?
This is the code example Microsoft provides:
using System;
class Class1
{
static void Main()
{
DateTime today = DateTime.Now;
DateTime answer = today.AddDays(36);
Console.WriteLine("Today: {0:dddd}", today);
Console.WriteLine("36 days from today: {0:dddd}", answer);
}
}
// The example displays output like the following:
// Today: Wednesday
// 36 days from today: Thursday
If DateTime.AddDays was a instance method, wouldn't something like DateTime.AddDays addDays = new DateTime.AddDays();
be required?
And, in general how should I think about static and instance variables? I've figured out that this is a tripping point for me, and I assume a very important concept.
Thanks in advance!
4 Answers
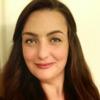
Jennifer Nordell
Treehouse TeacherEvan Welch, that's exactly correct! Here's an example I like to use. Let's say I have a zoo. In my zoo, I have some zebras. Each zebra is an instance of the Zebra
class. It has a name, age, weight etc. But that is specific to that zebra.
However, maybe I want to print out on a sign the average running speed of zebras as a whole and estimated to be 64 km/hour. If it were an instance method, I would have to have an instance of a zebra to print out the average running speed of all zebras. But that's sort of ridiculous. I should be able to print it out even if I currently have no zebras.
I would like to maybe be able to do something like, Cheetah.printAverageSpeed
, Zebra.printAverageSpeed
, Elephant.printAverageSpeed
. They should each still be closely tied to the class, because the average running speed of a cheetah is not the same as the average running speed as an elephant It's closely tied to the class, but not any specific instance of the class. 64 kilometers/hour on average applies to all zebras because it is all zebras on average. Not necessarily, "Fluffy" the zebra
Hope this helps!
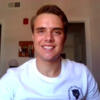
Evan Welch
1,815 PointsFunny answer and thank you.
So, any time I use instance variables or classes and methods, I must instantiate it before I can use it. However, with static methods and variables I don't need to instantiate, and I probably couldn't instantiate it even if I wanted to, right?

Simon Coates
8,484 PointsI don't think so. To instantiate is to create an instance.
(At some point, you may encounter 'static construction', but in that scenario, it doesn't mean instantiation. It's mostly just a way to set up static variables, which are, again, bound to the class. If you see it and it looks confusing, it doesn't change the basic static/instance divide.).
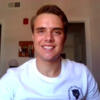
Evan Welch
1,815 PointsThat is helpful! And since I have you here, how would you go about coding printAverageSpeed
? Would you put it in a class of your own or would you define it as a method in some other class like the Zebra class or would you define it as a method in the Main function itself. I need a little help on the strategy since I want to practice writing code in a way that takes advantage of encapsulation.
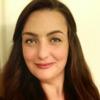
Jennifer Nordell
Treehouse TeacherEvan Welch I'd define it on the class Zebra. Each animal type would have its own. But imagine we had 150 animals. Each with a different top running speed. I suppose you could do a really long switch statement and say if the animal was a zebra, then print this, else if it was an elephant, print this other number. But it sure would be easier to just do Zebra.printRunningSpeed
, because it is not going to change for any particular instance of Zebra
. Rather, it applies to all zebras
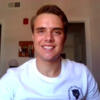
Evan Welch
1,815 PointsThank your for the answer Jennifer Nordell. So every animal I will need to know the average speed of will need to have a identical method printRunningSpeed
? That's totally fine if that's the best way to handle it, copy paste isn't too hard. I just don't want to feel like I'm hard coding something when there's a much more elegant solution available. Thanks again!
Simon Coates
8,484 PointsSimon Coates
8,484 PointsAn object has its own identity. So two dates have specifics that differentiate themselves from each other. Static methods or variables relate to the general concept - the class. And this is reflected in method calls. If the .MethodName follows an object, it's an instance method and if it's Class.MethodName, then it doesn't require access to anything particular to an instance. I'm completely butchering this. Um. today has an identity. AddDays uses on that identity to return a new instance of the datetime. And that's normal syntax for accessing an instance.
The concept is summarized at https://www.cis.upenn.edu/~matuszek/cit591-2006/Pages/static-vs-instance.html as "Classes declare instance variables and instance methods. Instance variables describe instances (particular objects). Instance methods work on instances. Classes can also declare static variables and static methods. Static variables are independent of any particular object. Static methods are independent of any particular object" (I'm including the link for purposes of citation. It's not in reference to C#, so reading the full article this might complicate things.)