Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial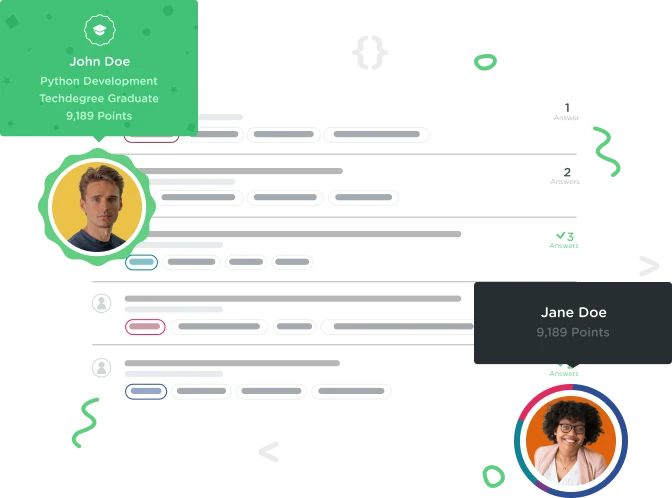
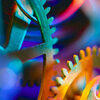
ja5on
10,338 PointsUp to two decimal places
Hi, in my code, one answer is 8.049999999999999 SqM I know I could reduce to 8.05 and that would be great because say it was a program for working out measurements. I have seen toFixed(2) but I'm at a loss as to where to insert that, or is there a different method to use?
Any help, thanks.
3 Answers

Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsOK, I see now, you need these `` around the template literal:
function getArea(width, height, unit) {
var area = width * height;
var a = area.toFixed(2);
return `${a} ${unit}`;
}
console.log(getArea(10.2, 20.495, 'sq ft'));
Output: 209.05 sq ft

Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsTry this:
var num = 8.049999999999999;
var n = num.toFixed(2);
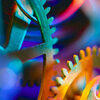
ja5on
10,338 PointsThat doesnt work with my code
function getArea(width, height, unit) {
var area = width * height;
var a = area.toFixed(2);
return ${area} ${unit}
;
}

Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsNot sure what your doing with the return part, but try this simple example first:
function getArea(width, height, unit) {
var area = width * height;
var a = area.toFixed(2);
return a;
}
console.log(getArea(10.2, 20.495, 10));
Output was 209.05
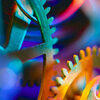
ja5on
10,338 Pointsreturn ${area} ${unit}
; Is a template literal. Without them I cannot access units.
ja5on
10,338 Pointsja5on
10,338 PointsYes I thought earlier that maybe on line var area = width * height; that maybe I could "get away" with adding .toFixed(2); I'm not against more lines of code, but I can see that line var area = width * height; has to declare the variable first.
Michael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsMichael Kobela
Full Stack JavaScript Techdegree Graduate 19,570 PointsWell, you could do these:
But I spaced it out early to try and explain your issue.