Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial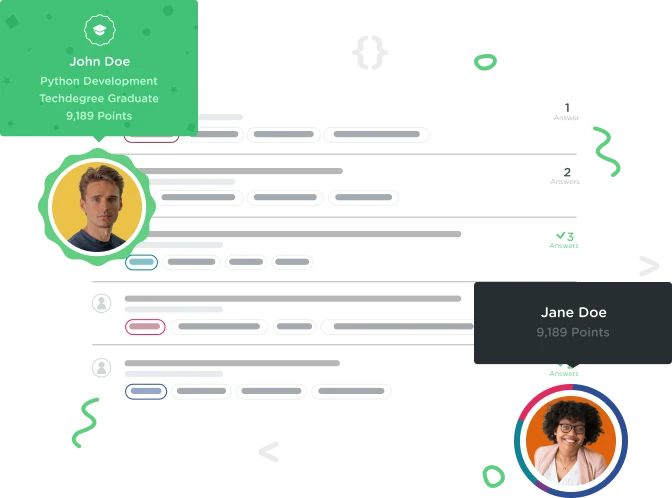
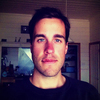
Sam Donald
36,305 PointsUse an array to check if $_GET["cat"] value is valid
Rather than write out a long if else
statement to check if the $_GET
value was valid and present I thought it would be more efficient to use an array.
<?php
// set default Page Title for catalog.php
$pageTitle = "Full Catalog";
// Build an array of excepted categories
$catArray = array("books", "movies", "music");
// Check to see if category is appended to url
if (isset($_GET["cat"])){
// Store the current category appended to the url
$currentCat = $_GET["cat"];
// Check if the $currentCat matches any value in the $catArray
foreach($catArray as $value){
if ($value == $currentCat){
// If it does set the $pageTitle to that value and capitalise first letters
$pageTitle = mb_convert_case($currentCat, MB_CASE_TITLE, "UTF-8");
} //end if
} //end foreach
} // end isset if
include("inc/header.php"); ?>
<div class="section page">
<h1><?php echo $pageTitle; ?></h1>
</div>
<?php include("inc/footer.php"); ?>
It looks long with the comments but I think its a lot more efficient. For example if you added more categories in the future all you'd have to do is add it to the end of the array. Rather than add all the extra else if
conditions like in the video.
i.e:
$catArray = array("books", "movies", "music", "games");