Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial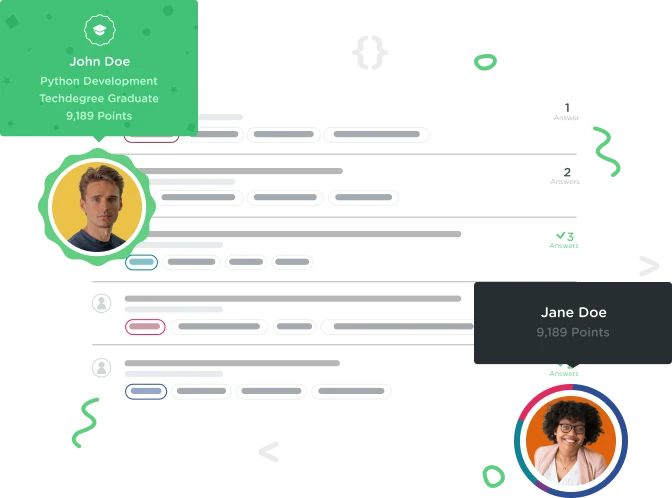

Jonathan McAlpine
1,812 PointsUsing console.printf to print out a formatted string
I am supposed to use console.printf to print out the response "Because you said <response>, you passed the test!"
I typed this code for one of the challenges and it came back with this response: "Did you use console.printf and to write out the formatted string with the response?"
Is there something missing or some better way I should write this?
1 Answer

qlpxjevhuv
10,503 PointsPersonally I would write something like this just to avoid declaring extra variables:
String response;
boolean tryAgain;
do {
response = console.readLine("Do you understand do while loops?");
tryAgain = response.equalsIgnoreCase("No");
if (!response.equalsIgnoreCase("No")) {
console.printf("Because you said %s, you passed the test!", response);
}
} while (response.equalsIgnoreCase("No"));
But the reason your code isn't passing the last part of the challenge is because you aren't utilizing the printf function.
String response;
boolean tryAgain;
// Removed boolean variable 'youPassed' since we aren't using it.
do {
response = console.readLine("Do you understand do while loops?");
tryAgain = response.equalsIgnoreCase("No");
if (!tryAgain) { //The '!' symbol means 'NOT'
// If NOT equal to value of 'tryAgain', execute if's code-block.
console.printf("Because you said %s, you passed the test!", response);
/* We are using printf function here which means 'print formatted.'
* So we want to display the response within our string.
* The %s means string, and we fill it with variable 'response' declared after our string.
*/
}
} while (response.equalsIgnoreCase("no"));
//Remove OR clause because we only want it to continue when the reply is "no."
//Removed: || response.equalsIgnoreCase("<response>")
Good job on the challenges so far and happy coding! Feel free to ask for help whenever you need it!
Jonathan McAlpine
1,812 PointsJonathan McAlpine
1,812 PointsOops, here is the code: