Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial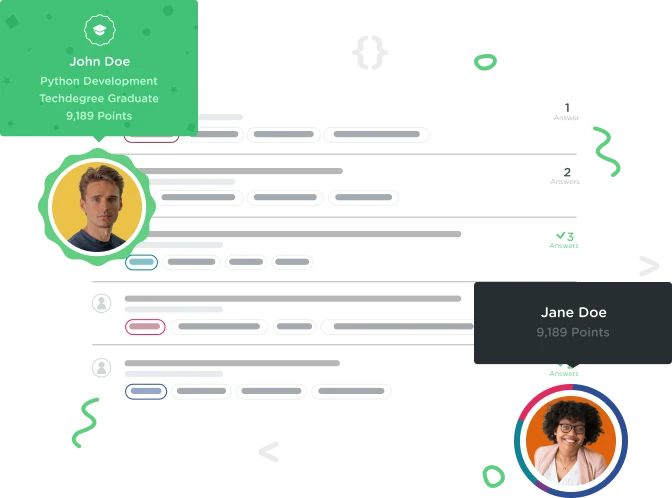

Gregory Serfaty
37,140 PointsValidation not correct
Why my condition is not valid ?
if ( fieldName.charAt(0) != 'm' && (! Character.isUpperCase(fieldName.charAt(1)))) {
throw new IllegalArgumentException('Error');
}

David B Dinkins
71,472 PointsCan either of you post the code that's working for you on this challenge? I've tried a bunch of different variations of the same code, and I'm coming up empty. Here's what I think is the best version of my answer:
public static String validatedFieldName(String fieldName) {
// Member fields must start with an 'm' and the second letter must be uppercased.
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
char fL = fieldName.charAt(0);
char sL = fieldName.charAt(1);
if (fL != 'm' && !Character.isLetter(sL) && !Character.isUpperCase(sL)) {
throw new IllegalArgumentException('Error');
}
return fieldName;
}
The only hint I'm getting is "Bummer! There is a compiler error. Please click on preview to view your syntax errors". But when I click on preview, the console is empty.
9 Answers

Gregory Serfaty
37,140 PointsDavid
You can have this name m_first_name so you need to check not the second letter but the third in this case.

David B Dinkins
71,472 PointsI'm still getting the same error. I hate to ask for a cheat, but I'm stuck and would understand better if I could see some code. Neither of these is working:
char fL = fieldName.charAt(0);
char sL = fieldName.charAt(1);
char tL = fieldName.charAt(2);
if (fL != 'm'
&& (!Character.isLetter(sL) || !Character.isLetter(tL))
&& (!Character.isUpperCase(sL) || !Character.isUpperCase(tL))) {
throw new IllegalArgumentException('Error');
}
char fL = fieldName.charAt(0);
char sL = fieldName.charAt(1);
char tL = fieldName.charAt(2);
if (fL != 'm' && !Character.isLetter(sL)
&& (!Character.isUpperCase(sL) || !Character.isUpperCase(tL))) {
throw new IllegalArgumentException('Error');
}

Craig Dennis
Treehouse TeacherWow looks like this is making things harder than needed.
Let me give you this hint, you can, and probably should, have 2 if
statements, and just throw 2 different exceptions.
You do not need to check the 3rd character. If the second character is an underscore, the field is named wrong. Just do these instructions:
// Member fields must start with an 'm' and the second letter must be uppercased.

Chase Marchione
155,055 PointsIn light of the new info, looks like this works:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// Member fields must start with an 'm' and the second letter must be uppercased.
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm') {
throw new IllegalArgumentException("Error");
} else if (!Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("Error");
} else {
return fieldName;
}
}
}
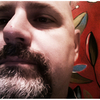
Jeremy Faith
Courses Plus Student 56,696 PointsYou can use an OR operator as Craig Dennis suggest and make it one line.
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("Error");
}

David B Dinkins
71,472 PointsAlright, that did the trick! I think I've been looking at that challenge the wrong way, because the comments in the method asked for the first letter to start "with an 'm' and the second letter must be uppercased". Anyways, huge help, thank you guys!
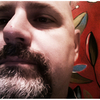
Jeremy Faith
Courses Plus Student 56,696 PointsI was stuck also until I saw Craig's suggestion. I am glad you got through the challenge.

Craig Dennis
Treehouse TeacherThanks for the feedback I'll update that comment so it's not misleading!

Gregory Serfaty
37,140 PointsOk thanks for your answer. It was the problem now all is fine ;)

Craig Dennis
Treehouse TeacherI think you want an OR don't you?

Gregory Serfaty
37,140 PointsDavid
try this
if(
(fieldName.charAt(0) != 'm') &&
(!Character.isLetter(fieldName.charAt(1)) && (!Character.isUpperCase(fieldName.charAt(2))))
||(!Character.isUpperCase(fieldName.charAt(1)))
)
{
throw new IllegalArgumentException("Error");
}
return fieldName;
}

Jess Sanders
12,086 Pointsprivate char validateGuess(char letter) {
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required."
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed") }
return letter;
}
The above code is the example to follow.
In pseudocode, we want to check for the following condition, and throw an error: " if the character at index 0 of fieldName does not equal 'm' OR the character at index 1 of fieldName is not upper case"
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("The field name does not match the style");
}
return fieldName;
}
}
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsI tried this challenge, and based on the errors received showing input strings, we need to check for the next letter after m which may or may not be at index 1.