Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial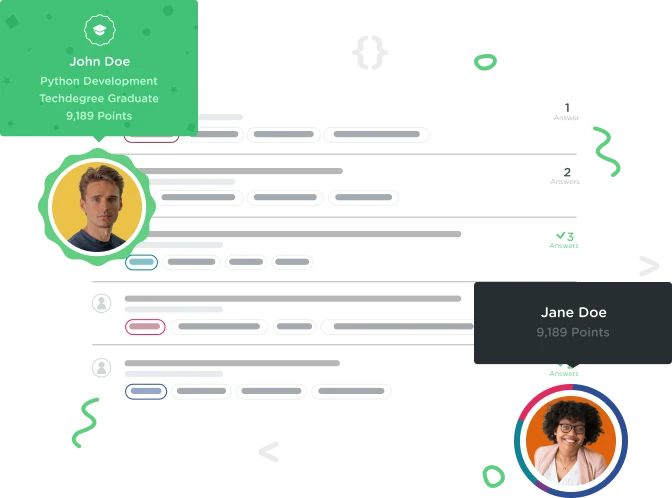

Mohammad Rifqi
Courses Plus Student 3,218 Pointsview generating random string
did anyone know how this code work ?and how to view this?
<?php
function str_rand($length =32, $character ='0123456789qwertyuiopasdfghjklzxcvbnmQWERTYUIOPASDFGHJKLZXCVBNM'){
if(!is_int($length || $character <0)){
return false;
}
}
$character_length = strlen($character) -1;
$string ='';
for($i =$length; $i >0; $i--){
$string .= $character[mt_rand(0, $character_length)];
return $string;
}
?>
3 Answers
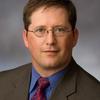
Ted Sumner
Courses Plus Student 17,967 PointsYou have a whole lot going on with this code that I think is problematic. The basic idea of a function is to do something when called by the main code. Variables in the name of the function is how to pass information into the function. The function has to return something that is then used in the main program. So the format would be something like this:
<?php
function test($var) {
// your code here, like
echo "Hello, $var);
}
$call = test("ted");
echo $call;
// Returns: Hello, ted
You do not have a call to the function.
In your if statement, you need something like this: ($length > 0 || $character < 0)
. You are missing something for the first variable.
This line is unusual: $character[mt_rand(0, $character_length)];
. I don't think it is correct, but would have to research it.
I am not sure if strlen()
is a built in function. If it is, check the documentation to see if it is correct. If not, it is undefined.
This should get you well on your way to functional code. Let me know if I can help more. I would need a better idea of what you want to accomplish.

Greg Kaleka
39,021 PointsHey Ted,
Corrections for your example:
<?php
function test($var) {
// your code here, like
return "Hello, $var";
}
$call = test("ted");
echo $call;
// Returns: Hello, ted
Cheers,
-Greg

Greg Kaleka
39,021 PointsHi Mohammad,
I also see a few errors in this code: the is_int call needs a closing parenthesis and the character check needs to check strlen($characters) and check greater than zero, not less than zero, the return statement needs to be outside the for loop and the function is closed in the wrong place. Here's a corrected version:
<?php
function str_rand($length = 32, $character = '0123456789qwertyuiopasdfghjklzxcvbnmQWERTYUIOPASDFGHJKLZXCVBNM')
{
// fixed parentheses, added length>0 check and changed to &&s
if(!(is_int($length) && $length > 0 && strlen($character) > 0))
{
return false;
}
$character_length = strlen($character) - 1;
$string ='';
for($i = $length; $i > 0; $i--){
$string .= $character[mt_rand(0, $character_length)];
}
return $string;
} // closing function here
// call the function and store the result in a variable
$random_string = str_rand();
// spit out the value of the variable to the screen when you load this file
var_dump($random_string);
?>
Here's what the function does, as I read it:
Essentially, the function is meant to choose random characters from the character set provided, and creates a string using those characters. The function then returns the string.
The function, str_rand
, takes two arguments; a length, and a string of characters. Both arguments have default values. The length is 32 unless specified otherwise, the string of characters is a string consisting of 0-9, a-z and A-Z.
The function checks to make sure the two arguments were supplied correctly (the length should be an integer, and the character set should have at least one character in it), and if not it returns false
.
If the check is passed, an empty $string
is initialized, and a for loop adds a random character from the character string provided (or the default char string) to the $string
. The line $character[mt_rand(0, $character_length)]
picks a random integer between 0 and the length of the character string minus one (since a string is a 0-based array of characters) and indexes into the string to pull out that character. This loop runs until the string is the length provided in the function call (32 characters long by default).
Then the function returns the string that's been built up.
To display the result on the screen, you need to call the function and then var_dump it, or use it some other way to display it. I've used var_dump in my example above.
Let me know if you have any other specific questions!
Cheers,
-Greg
P.S. - A quick word about spacing. PHP doesn't care about this, per se, but it's a best practice to keep your spacing "balanced" around mathematical operators. In almost every instance of a mathematical operator you put a space before but not after it. That makes it harder to read. Either don't use spaces or do, but keep them balanced. Personally I strongly prefer spaces.
$var = 2 + 5;
for example, not $var =2 +5
.
Some languages actually enforce this as a rule (though as I said PHP is not one of them).
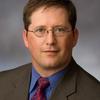
Ted Sumner
Courses Plus Student 17,967 PointsI have looked at your code more and have some more comments but would like to refine them. What are you trying to accomplish with this code?
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 Pointsformatted code quote. You had it correct except adding the language after the first three `. There should be no space between the ` and the language.