Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial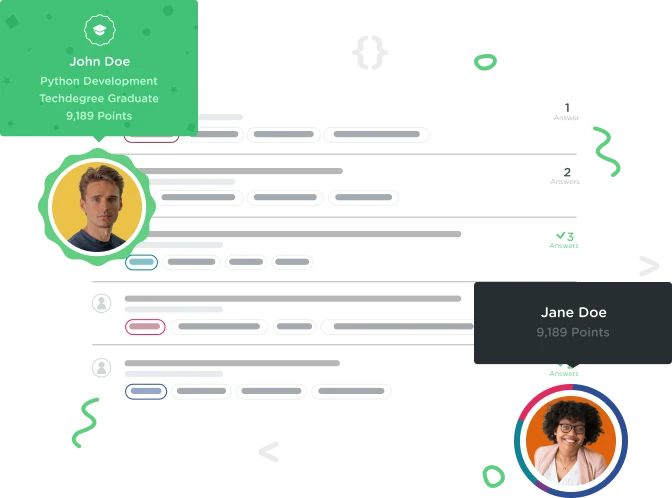
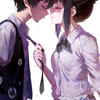
olliecee2
1,550 PointsWebpack, React and vendor JavaScript libraries
I'm trying to figure out how to add 3rd party JavaScript libraries into my React application and bundling them into .js file.
The JS libraries I'm using are: jQuery, Tether, Bootstrap 4 and wowjs.
Note: There is a load sequence for Bootstrap to work. jQuery > Tether > Bootstrap 4
I've tried two methods so far.
I used both 'import' and 'require' in my entry file to add my JS files but they never bundled in the correct order. Bootstrap would always load before jQuery and Tether, causing issues cause it depends on those to be loaded first.
I used the 'CommonsChunkPlugin' which is a Webpack plugin. It basically just creates a separate JS file that contains all your JS vendor files. The issue here is the same. It always bundles my JS libraries in the incorrect order.
Both of these methods always bundle my JavaScript libraries in: Bootstrap, jQuery, Tether and wowjs. The correct sequence is jQuery, Tether, Bootstrap, wowjs.
Can anyone figure out why Webpack is bundling my JS libraries in this odd order?
Here is my webpack.config.js file for method 2.
module.exports = {
entry: {
app: "./app.jsx",
vendor: [
"jquery",
"tether",
"bootstrap",
"wowjs"
]
},
output: {
path: path.resolve(__dirname, "build"),
filename: "bundle.js"
},
module: {
loaders: [
{
test: /\.jsx$/,
loaders: ['uglify-loader', 'babel-loader'],
exclude: [
'/node_modules/',
'/build/'
]
}
]
},
plugins: [
new webpack.optimize.CommonsChunkPlugin({
name: "vendor",
filename: "vendor.bundle.js"
}),
new webpack.optimize.UglifyJsPlugin(),
]
};
There are two important things happening here:
- Webpack creates a bundle.js file containing my React application. Totally fine.
- Webpack creates a vendor.bundle.js file containing my 3rd party JS libraries. Seems OK
The issue I have here is that we all know that Bootstrap 4 can't work without jQuery and Tether being included first. After I run webpack to build my assets, it spits out these two files. After the files have been generated, I view the vendor.bundle.js through my IDE only to find that the order they're bundled is: Bootstrap 4 > jQuery > Tether > wowjs
I don't think I have to mention the console logged errors. They're self explanatory, "Bootstrap's JS requires jQuery. jQuery must be included before Bootstrap's JS". Clear as day.
Does anyone know how to solve this or know any other solutions in creating a bundled .js file with vendor libraries in a specific order?