Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial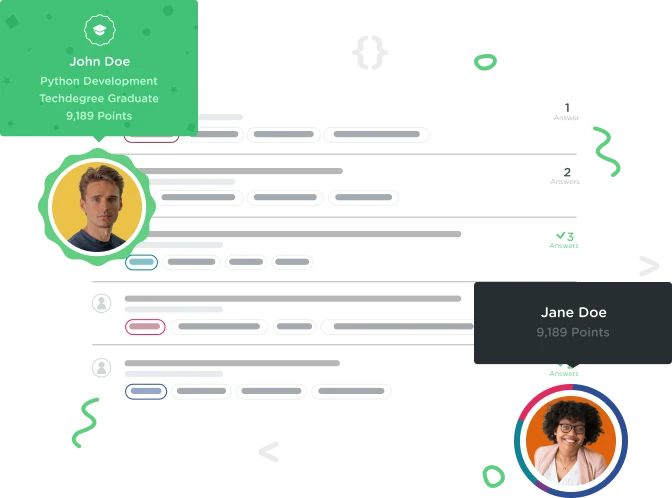

William J. Terrell
17,403 PointsWell something is broken...
When trying to compile in the console, I get this error:
Example.java:6: error: constructor PezDispenser in class PezDispenser cannot be applied to given
types;
PezDispenser dispenser = new PezDispenser();
^
required: String
found: no arguments
reason: actual and formal argument lists differ in length
1 error
My code is as follows:
// Example.java
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser();
System.out.printf("The dispenser character is %s\n",
dispenser.GetCharacterName());
}
}
//PezDispenser.java
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean isEmpty() {
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String GetCharacterName() {
return mCharacterName;
}
}
Any assistance would be appreciated :)
Thanks!
1 Answer

Milo Winningham
Web Development Techdegree Student 3,317 PointsIn Example.java you're missing the characterName
argument when creating the new PezDispenser
. Your REPL example works because you provide "William" to the constructor.

William J. Terrell
17,403 PointsSo it's not really "broken", it just hasn't been defined yet?

Milo Winningham
Web Development Techdegree Student 3,317 PointsNope, you do need to fix your code in Example.java since the PezDispenser
class is defined as requiring a characterName
when it is instantiated.
The error message is a little cryptic but it says essentially the same thing: new PezDispenser()
won't work because a String
argument is required. In the REPL, new PezDispenser("William")
works because you've provided a String
argument of "William".

William J. Terrell
17,403 PointsSo it has to have a default value defined, then? I tried doing it that way (since in the video Craig has "Yoda" defined as a default value, at least that's my understanding of it), but I still get errors...
From what I can tell, my code is exactly the way it is in the video (except I have "Default" in place of "Yoda"):
//Example.java
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser("Default");
System.out.printf("The dispenser character is %s\n",
dispenser.GetCharacterName());
if (dispenser.isEmpty) {
System.out.println("It is currently empty");
}
System.out.println("Loading...");
dispenser.load();
if (!dispenser.isEmpty) {
System.out.println("It is no longer empty! :)");
}
}
}
//PezDispenser.java
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean isEmpty() {
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String GetCharacterName() {
return mCharacterName;
}
}
//This is the error I am currently getting, when I compile Example.java:
Example.java:9: error: cannot find symbol
if (dispenser.isEmpty) {
^
symbol: variable isEmpty
location: variable dispenser of type PezDispenser
Example.java:9: error: illegal start of type
if (dispenser.isEmpty) {
^
Example.java:14: error: cannot find symbol
if (!dispenser.isEmpty) {
^
symbol: variable isEmpty
location: variable dispenser of type PezDispenser
3 errors

William J. Terrell
17,403 PointsActually, I think I see what is wrong here: In Craig's Example.java, he has:
if (dispenser.isEmpty())
And in mine, it is just:
if (dispenser.isEmpty)
I fixed that and the console reads as follows when Example.java is compiled:
We are making a new Pez Dispenser.
The dispenser character is Default
It is currently empty
Loading...
It is no longer empty! :)
So, that being said, just so I better understand how things work, why does isEmpty need the double-parentheses () after it? (is it because it is functioning as a method of dispenser?)

Milo Winningham
Web Development Techdegree Student 3,317 PointsRight, you need parentheses after isEmpty
because it is a method.
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsIt does seem to be working in REPL, though. The console is as follows: