Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial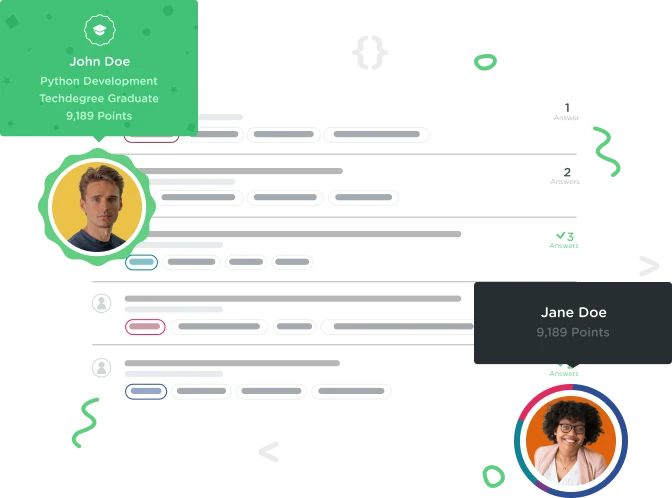

Solomon Alexandru
3,633 PointsWhat am I doing wrong?
I'm struggling for a while with this exercise and I can't seem to get past it
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
while (mBarsCount != MAX_ENERGY_BARS) {
return mBarsCount++;
}
}
}
2 Answers
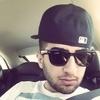
Vrezh Gulyan
11,161 PointsFirst of all reset the code to what it was before this challenge. Remove
while (mBarsCount != MAX_ENERGY_BARS) {
return mBarsCount++;
}
Next what the challenge is asking you to do is to increment the number of energy bars as long as the battery is not fully charged. This is really easy since you just made a helper method called isFullyCharged which returns true once the battery level == 8 which is the max level. All you have to do is keep checking that isFullyCharged method and if it isnt full then you keep adding(charging) to the battery level. Since you are going to keep doing the same thing over and over you should see immediately that this is the job for a loop. So your charge method should now look like this:
public void Charge() {
// Will return false until battery is full
while( isFullyCharged == false){
//increment bars
mBarsCounts++;
}
}
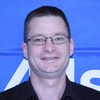
Seth Reece
32,867 PointsHi Solomon,
Two thing here. I believe you want your while loop inside the charge method. Second the challenge is asking to use the isFullyCharged method. You loop should be structured:
while (!isFullyCharged()) {
mBarsCount++;
}

Solomon Alexandru
3,633 PointsStill not working
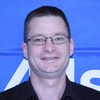
Seth Reece
32,867 PointsHere's the full passing code:
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}