Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial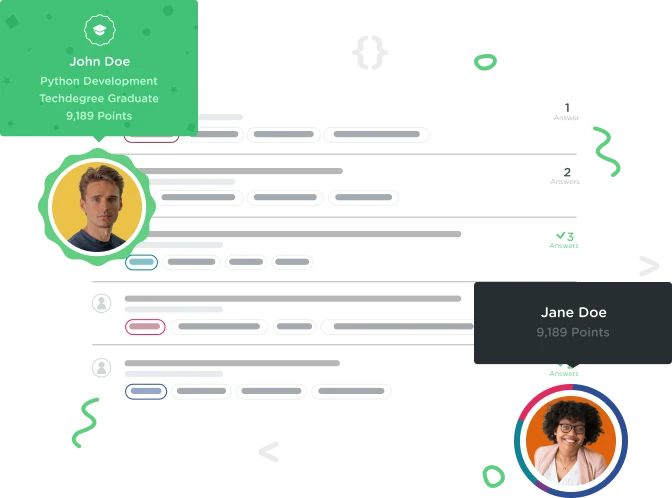

Thea Partin
8,750 PointsWhat am I doing wrong?
using System;
class Program
{
static double Multiply(double firstParam, double secondParam)
{
console.writeline ( firstParam * secondParam);
}
static void Main(string[] args)
{
double add1 = Multiply(2.5, 2);
Console.WriteLine(add1);
double add2 = Multiply(6,7);
Console.WriteLine(add2);
Console.WriteLine(add1 + add2);
}
}
3 Answers
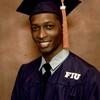
Dane Parchment
Treehouse Moderator 11,077 PointsThis is not the correct answer! I didn't realize the prompt had a challenge associated to it and only answered the question based on the code provided. I'll keep this answer though because it does address what's wrong with the code given.
Your function is expecting to return a double but you aren't actually returning any values. Syntax structure aside, your code here:
class Program
{
static double Multiply(double firstParam, double secondParam)
{
console.writeline ( firstParam * secondParam);
}
Should be doing this:
class Program
{
static double Multiply(double firstParam, double secondParam)
{
return firstParam * secondParam;
}
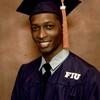
Dane Parchment
Treehouse Moderator 11,077 PointsHaving looked at the challenge itself I realize I have given you the wrong answer as I was only looking at the code you provided, not at what the challenge itself asked you to do. My bad, I'll keep the original answer up for posterity's sake.
Now as for the problem: You are tasked with creating a static method that will multiply two doubles together and write the result to the console. You almost had it.
For starters your syntax is wrong for the method definition, they explicitly ask for a void method, not a double, because you won't be returning any values.
Next you need to capitalize and camelcase the function call for console.writeline
=> Console.WriteLine
Finally in the main method you are assigning the multiply method calls to variables, which you shouldn't be doing because not only is that not what the prompt asked for, but you also aren't returning any values from the method so there is nothing to assign.
Here is the total fix:
using System;
class Program
{
// YOUR CODE HERE: Define a method named Multiply. Remember
// to use the "static" and "void" keywords: "static void
// Multiply" (without quotes). Multiply should take two
// "double" values as parameters.
static void Multiply(double firstNumber, double secondNumber) {
Console.WriteLine(firstNumber * secondNumber);
}
static void Main(string[] args)
{
// YOUR CODE HERE:
// Call Multiply with two arguments: 2.5 and 2.
Multiply(2.5, 2);
// YOUR CODE HERE:
// Call Multiply with two arguments: 6 and 7.
Multiply(6, 7);
}
}