Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial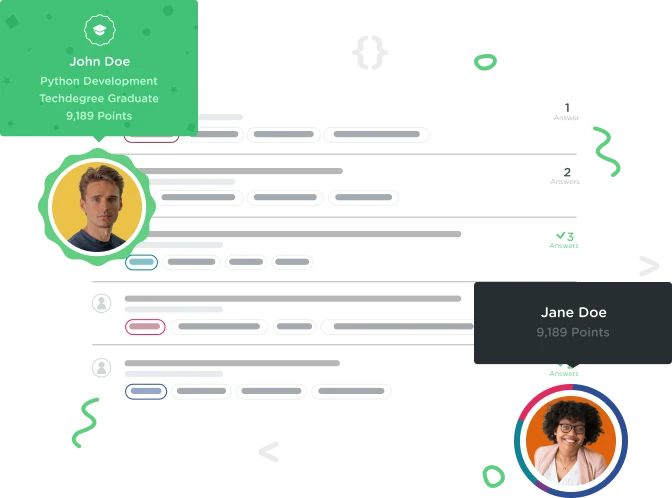

Jorleni Maldonado
14,398 PointsWhat am I missing?
Please help :)
var birds = new List<Bird>
{
new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 },
new Bird { Name = "Dove", Color = "White", Sightings = 2 },
new Bird { Name = "Robin", Color = "Red", Sightings = 5 },
new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 },
new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 },
new Bird { Name = "Crow", Color = "Black", Sightings = 11 },
new Bird { Name = "Pidgeon", Color = "White", Sightings = 10 }
};
var anyBlueBirds = false; if birds.Any(b=>b.Color == "Blue"); { anyBlueBirds = true; }
var allBlueBirds = false; if birds.All(b=>b.Color == "Blue"); { allBlueBirds = true; }
1 Answer
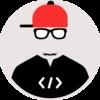
Luis Duenas
5,967 PointsBoth of your answers are wrong, but let me focus in this line
var allBlueBirds = false; if birds.All(b=>b.Color == "Blue"); { allBlueBirds = true; }
here you are saying "set allBlueBirds to false, then evaluate if all birds are blue then set allBlueBirds to true"
var allBlueBirds = false; // set variable to false
if birds.All(b=>b.Color == "Blue"); // evaluate if all birds are blue but do nothing because of the semicolon
{ allBlueBirds = true; } // set variable to true
when you put the semicolon at the end of the if statement, the code inside the following brackets doesn't care for that evaluation, also, as shown in the videos, you can simply assing the value of the linq expression to your variable like this:
var anyBlueBirds = birds.Any(b => b.Color == "Blue");
var allBlueBirds = birds.All(b => b.Color == "Blue");
Jorleni Maldonado
14,398 PointsJorleni Maldonado
14,398 PointsCreate a variable named allBlueBirds and write a LINQ query using the All method to assign a boolean value if all of the Bird objects in the birds list have the value "Blue" in the Color property.