Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial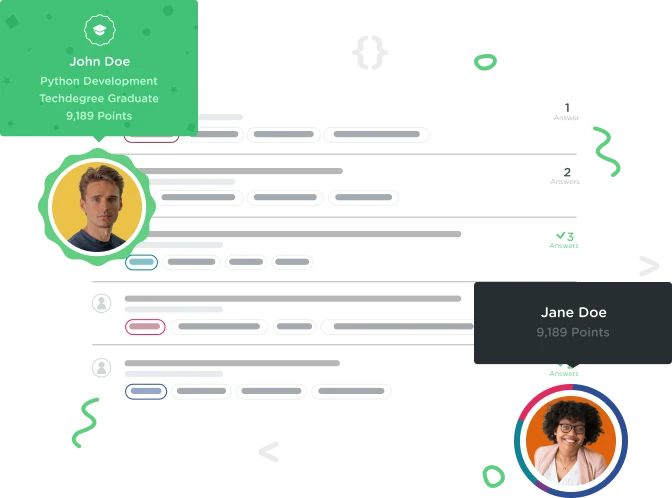
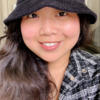
Eunice Oquialda
1,357 PointsWhat did I do wrong? Pleaase see my code:
// 1. Declare variables and capture input. const adjective = prompt('Please type an adjective.'); const verb = prompt('Please type a verb'); const noun = prompt('Please type a noun.');
// 2. Combine the input with other words to create a story
const sentence = <p>There once was a ${adjective} programmer who wanted to use JavaScript to ${verb} the ${noun.} </p>
;
// 3. Display the story as a <p> inside the <main> element. document.querySelector('main').innerHTML = sentence;
Reference: Variables and Strings Challenge Solution video on JS basics video time: 5:32
3 Answers
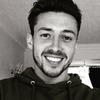
Shaun Kelly
35,560 PointsHi Eunice,
I have just spotted that you have a period inside of your noun literal. It should read ${noun}. Rather than ${noun.}
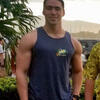
Jonah Poulson
Python Development Techdegree Graduate 32,616 PointsIt's either your missing the backticks in your template literal or you didn't target the main element right as you are missing the class or id selector in your document.querySelector() method
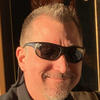
Peter Vann
36,427 PointsHi Eunice!
I agree with Jonah.
I think your main issue is that your string/template literal needs to be surrounded by backticks (`) (The key above the 'Tab' key)
This works:
// 1. Declare variables and capture input.
const adjective = prompt('Please type an adjective.');
const verb = prompt('Please type a verb');
const noun = prompt('Please type a noun.');
// 2. Combine the input with other words to create a story
const sentence = `There once was a ${adjective} programmer who wanted to use JavaScript to ${verb} the ${noun}.`;
// 3. Display the story as a <p> inside the <main> element.
document.querySelector('.main').innerHTML = '<p>' + sentence + '</p>';
This is assuming that the "main" element has the class "main". If it has the id of "main', then you would need the code for the third step to be:
document.querySelector('#main').innerHTML = '<p>' + sentence + '</p>';
Keep in mind that document.querySelector() requires the '.' or the '#' to target the right element, whereas something like document.getElementById() or document.getElementsByClassName() do not (just the name without the id/class identifier).
This also works just as well:
// 1. Declare variables and capture input.
const adjective = prompt('Please type an adjective.');
const verb = prompt('Please type a verb');
const noun = prompt('Please type a noun.');
// 2. Combine the input with other words to create a story
const sentence = `<p>There once was a ${adjective} programmer who wanted to use JavaScript to ${verb} the ${noun}.</p>`;
// 3. Display the story as a <p> inside the <main> element.
document.querySelector('.main').innerHTML = sentence;
More info:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals
I hope that helps.
Stay safe and happy coding!
Eunice Oquialda
1,357 PointsEunice Oquialda
1,357 PointsOh snap! I didn't see that!! Thank you!