Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial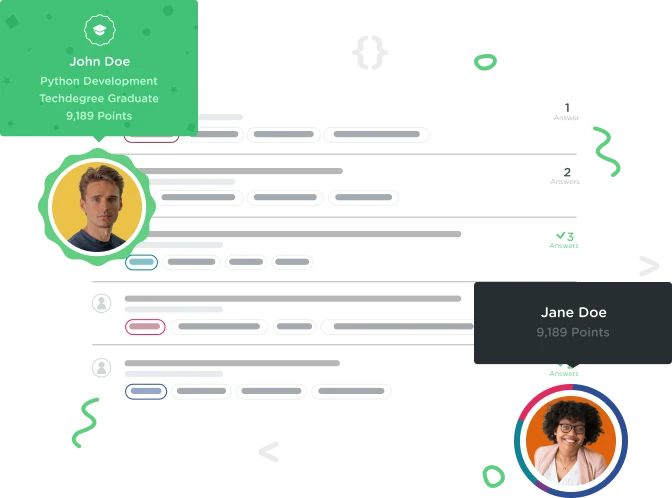
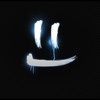
Grigorij Schleifer
10,365 PointsWhat i am doing wrong?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
this is the code i put in to solve the challenge
public int getTileCount() {
for (char character: mHand.toCharArray()){
if (mHand.hasTile()) {
mHand.addTile();
}
mHand.getTileCount();
}
i get this errors
./ScrabblePlayer.java:20: error: illegal start of expression
public void addTile(char tile) {
^
./ScrabblePlayer.java:20: error: illegal start of expression
public void addTile(char tile) {
^
./ScrabblePlayer.java:20: error: ';' expected
public void addTile(char tile) {
^
./ScrabblePlayer.java:20: error: ';' expected
public void addTile(char tile) {
^
./ScrabblePlayer.java:25: error: illegal start of expression
public boolean hasTile(char tile) {
^
./ScrabblePlayer.java:25: error: ';' expected
public boolean hasTile(char tile) {
^
./ScrabblePlayer.java:25: error: ';' expected
public boolean hasTile(char tile) {
^
./ScrabblePlayer.java:28: error: reached end of file while parsing
}
^
./ScrabblePlayer.java:14: error: cannot find symbol
if (mHand.hasTile()) {
^
symbol: method hasTile()
location: variable mHand of type String
./ScrabblePlayer.java:15: error: cannot find symbol
mHand.addTile();
^
symbol: method addTile()
location: variable mHand of type String
./ScrabblePlayer.java:17: error: cannot find symbol
mHand.getTileCount();
^
symbol: method getTileCount()
location: variable mHand of type String
./ScrabblePlayer.java:25: error: variable tile is already defined in method getTileCount()
public boolean hasTile(char tile) {
^
./ScrabblePlayer.java:26: error: incompatible types: boolean cannot be converted to int
return mHand.indexOf(tile) > -1;
^
13 errors
6 Answers
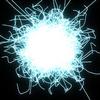
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello,
Looks like you're on the right track with this, just needs a little bit of help.
You first want to make sure you pass in a char argument in your getTile() count, this is the char that we want the method to check for.
Also before you begin your loop be sure to create a int count variable so that we can count how many times the character passed in the argument is found in mHand once we make it a char array.
Once these steps are down you'll use a for loop to go through each char in mHand Array as you did, but instead check to see if the current letter is equal to the character you passed in as the argument, and increment your count by one if it is.
Finally once out of your for and if statement return your count variable
In short it should look something similiar to
public int getTileCount(char tile) {
int count =0;
for (char letter : mHand.toCharArray()) {
if (letter == tile) {
count++;
}
}
return count;
}
Thanks, feel free to shout if this doesn't help.

Mark VonGyer
21,239 PointsCount your open brackets and closed brackets.
You haven't closed them all off correctly.
public int getTileCount() { for (char character: mHand.toCharArray()){ if (mHand.hasTile()) { mHand.addTile(); } mHand.getTileCount(); }
Here i saw 3 open brackets, 2 closed brackets.
Therefore there is a bracket missing. This is the cause of most your errors.
public boolean hasTile(char tile) { return mHand.indexOf(tile) > -1; }
This part is also wrong.
You have declared a boolean should be returned (which seems correct), however you are returning an integer(and then you have an illegal expression).
public boolean hasTile(char tile){ if(mHand.indexOf(tile)>-1){ return true; } else{ return false; } }
Finally:
public int getTileCount() { for (char character: mHand.toCharArray()){ if (mHand.hasTile()) { mHand.addTile(); } mHand.getTileCount(); }
This code is a method which calls itself, creating an endless loop. It also has no return statement. You need to return an integer.
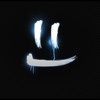
Grigorij Schleifer
10,365 PointsNow i get a single error
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount() {
int tileCount = 0;
if (mHand.hasTile()) {
tileCount ++;
}
return tileCount;
}
}
This is teh error :
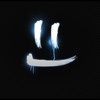
Grigorij Schleifer
10,365 PointsNow i get a single error
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount() {
int tileCount = 0;
if (mHand.hasTile()) {
tileCount ++;
}
return tileCount;
}
}
This is the error i get:
./ScrabblePlayer.java:23: error: cannot find symbol
if (mHand.hasTile()) {
^
symbol: method hasTile()
location: variable mHand of type String
1 error
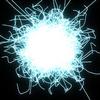
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello again, you don't need to use the mHand.hasTile() method, just loop through each char in a for loop and compare it to the the char you passed in the argument of the getTileCount.
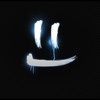
Grigorij Schleifer
10,365 PointsGreat !!!! It is working :) But i have another Question. Why i have to declare "char tile" in the () again ? I have it in the hasTile method ... confused

Mark VonGyer
21,239 PointsYou have created two different methods - each one takes a parameter. You need to declare what parameter type you are going to use and then give it a name.
So basically you are saying. I want to create a method that will add a tile. Now I want to give that method a CHARACTER (primitive data type - char), and I will call that char tile. Please remember this char everytime I refer to it in this method by the nick name I have given it.
You could quite have easily said
public void addTile(char lolThisIsACrazyParameterTryToFigureThisOutCompiler){}
to the compiler it makes no difference - but you have used char tile because it makes sense - you are passing a tile to it. That way if I read your code it makes sense to someone else.
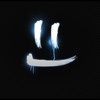
Grigorij Schleifer
10,365 PointsThanks a lot Mark ... and i promise u , i will never stop trying to crash the damn Compiler !!!!