Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial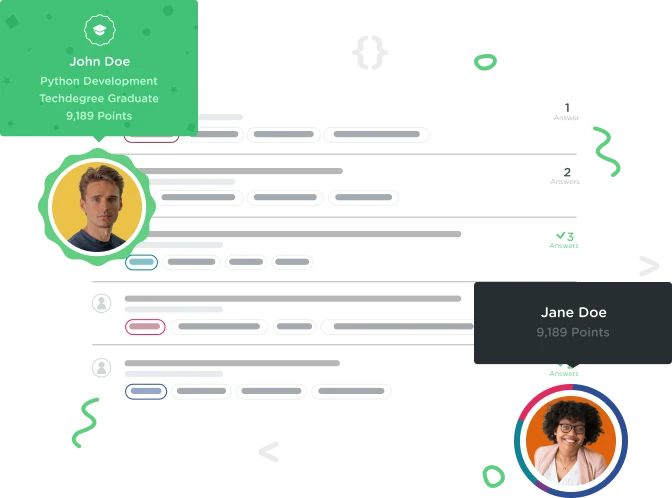

Phuong-Lan Nguyen
1,764 PointsWhat is the difference between a parameter and an argument? How are they related to each other?
I'm getting the two confused. I've googled around, but none of them are very clear..
This task is to "pass an argument to a function" -- I feel like what I did in my script was call up (return) a parameter?
function returnValue(drink){
return drink;
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
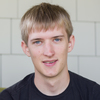
krilnon
1,458 PointsIn computer science, there are two kinds of parameters: formal parameters and actual parameters. Actual parameters are the same thing as arguments.
Formal parameters are the identifiers in a function declaration, like a
and b
here:
function example(a, b){
// do stuff
}
var c = 2, d = 5
example(c, d)
c
and d
are the arguments: the actual parameters.
To make an analogy, (formal) parameters are the guest list, and arguments are the list of people who actually showed up to the function. ('Function' has a dual meaning in this analogy... it can be a function like a party, or a function like a JavaScript function/procedure.)
This task is to "pass an argument to a function" -- I feel like what I did in my script was call up (return) a parameter?
In task 1, within the function you define, you're returning a parameter. In task two, your job is to call that function with an argument. So what you feel you did is correct. In task 1, you're setting up rules so that eventually a parameter will be returned, and in task 2 you're carrying out the function call so that the parameter is actually returned.
Phuong-Lan Nguyen
1,764 PointsPhuong-Lan Nguyen
1,764 Pointsthat makes a lot of sense, thank you!!
I realized I have to think of parameters as variables (but they're within a function, rather than the variables we define as "var"). And arguments are like the actual equations that you're trying to compute.
When I'm "passing an argument to a function" I'm specifying the values for those variables (parameters) so that the equation (arguments) can be computed.
..Is that right? I come from a math/science background so it makes more sense to me to think in those terms.
krilnon
1,458 Pointskrilnon
1,458 PointsIt sounds like you understand things pretty well. One thing that sticks out to me is when you say "equation". Usually in a procedural language like JavaScript, you are only ever computing one side of an equation at a time.
So you kind of need to formulate everything as
y = (5 * (x - 1)) / x
rather thany + x * 5 - x * y = y + 5
, because you have to figure out the structure for how to solve the equation, JavaScript just handles the part where it substitutes the variables for values.Hope that helps; I think you've already got it.