Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial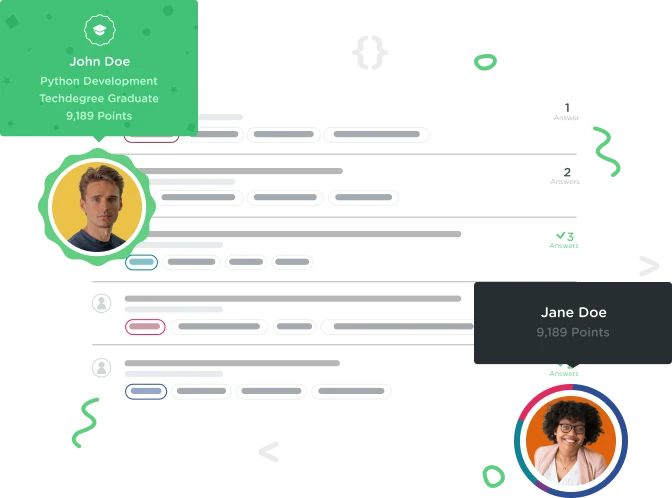

Erik Luo
3,810 PointsWhat is the normal way to complete this challenge?
I completed this challenge by searching on google and finding methods, but I didn't use a loop. I tried to use a loop but didn't work. Can someone tell me the how to complete this challenge in the way it intended to?
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
// Prompts the user to enter the number of times to enter “Yay!”. I've done this for you. Leave this as it is.
Console.Write("Enter the number of times to print \"Yay!\": ");
// Prints “Yay!” to the screen that number of times
int num_1 = Convert.ToInt32(Console.ReadLine());
var Yay_num = String.Concat(Enumerable.Repeat("Yay!", num_1));
Console.WriteLine(Yay_num);
}
}
}
2 Answers

andren
28,558 PointsI'm actually pretty surprised the challenge accepted that as a valid answer, the challenge checking code tends to be pretty picky about what solutions it accepts even if they produce the right output, and your code is very different from the intended solution.
The intended solution is to use a while loop like this:
Console.Write("Enter the number of times to print \"Yay!\": ");
// Get the input string and convert it to an int using int.Parse
int timesToRun = int.Parse(Console.ReadLine());
// declare a counter variable that will be used to keep track of the number of times the loop has ran
int counter = 0;
// Run the loop while the counter is less than the timesToRun variable
while(counter < timesToRun) {
// Print "Yay!"
Console.Write("Yay!");
// Increase the counter variable by 1
counter++;
}
This is the solution you were meant to write based on the things you have been tough in the course so far. You can also use a for loop to do this more efficiently, but If I remember correctly for loops have not been introduced yet by the time you get to this challenge.

Stephan Olsen
6,650 PointsYou would save the input from the user in a variable and then convert it to Int, as you're already doing. After that you would use it is a condition in a foor loop.
for(int i = 0; i < num_1; i++)
{
Console.WriteLine("Yay!");
}