Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial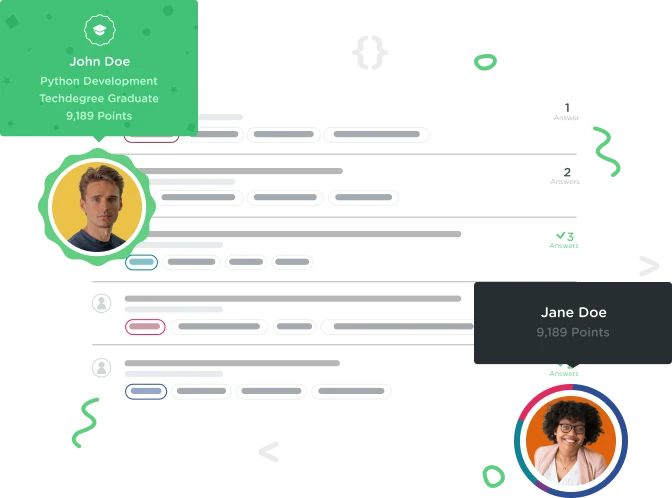
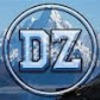
Liam Andersson
1,396 PointsWhat is the problem with this code? | c#
I basically want to create an int that generates random numbers. I also want to use it in a different class. Does anyone know how I can do that?
My code:
class Random
{
Random rnd = new Random();
public int num01 = rnd.Next(1, 20);
public int num02 = rnd.Next(1, 10);
}
1 Answer

Shadab Khan
5,470 PointsHi Liam, Assuming your lower bound is 1 and upper bound is 20, you could use a static class like so
namespace WhateverYourAppNameSpaceIs
{
static class Random
{
public int Next()
{
System.Random rnd = new System.Random();
return rnd.Next(1, 20)
}
}
}
The above technique of using a static Random class neatly refactors a random number generator for your application. Now, whenever you wish to generate a random number from anywhere in your client / consuming code all you need to do is write this statement :
int random = Random.Next();
Pay close attention as I don't need to create a random class object every time I need a random number generated; I am calling the Next() method on Random class directly that I have created within the app's namespace.
Hope that helps, and happy coding :)