Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial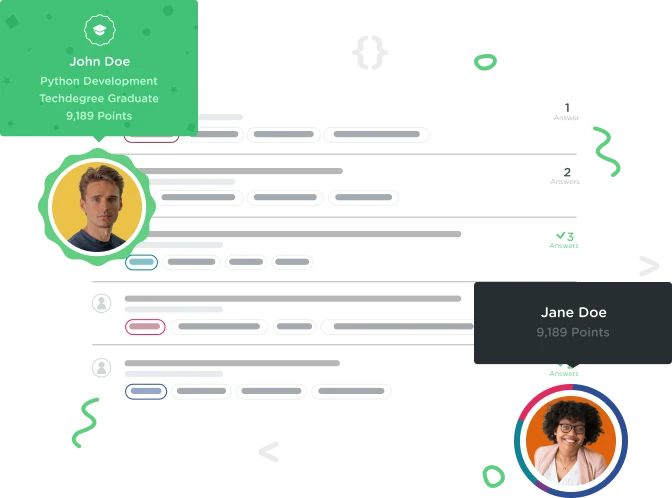

Barry Ort
12,373 PointsWhat is wrong?
I get an error that task #1 isn't working, but when I go back to task #1 everything works fine. So why is this not working?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var counter = 0;
var amount = 0;
while(counter < amount) {
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
amount = int.Parse(entry);
Console.WriteLine("Yay!");
counter++;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
2 Answers

Samuel Savaria
4,809 Points"var amount = int.Parse(entry);" needs to be inside the "try". The try and catch method is used to prevent exceptions from crashing your program. If "catch" detects an exception, it will destroy the value and run whatever code you want.
You are using the .Parse method. The problem with it is, if the use enters a string, the program will crash. So, you use the try and catch to prevent it. If any code in "try" gets the exception "FormatException", the program will destroy the string value and run "catch" instead.

Samuel Savaria
4,809 PointsI'm not sure what you mean by task, but you should the prompt the user to enter the number of times to print "Yay", before the loop. As it is now, you are asking the user how many times to print "Yay", print it once, and ask again.
Also, you could optimise your code by keeping the .Parse method out of the loop. You only need to ask for the number of times and convert it to an integer once.

Barry Ort
12,373 Pointshmmm... I changed the code and it still isn't working
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
var amount = int.Parse(entry);
var counter = 0;
while(counter < amount) {
try
{
Console.WriteLine("Yay!");
counter++;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}

Samuel Savaria
4,809 Points"var amount = int.Parse(entry);" needs to be inside the "try". The try and catch method is used to prevent exceptions from crashing your program. If "catch" detects an exception, it will destroy the value and run whatever code you want.
You are using the .Parse method. The problem with it is, if the use enters a string, the program will crash. So, you use the try and catch to prevent it. If any code in "try" gets the exception "FormatException", the program will destroy the string value and run "catch" instead.
I hope you get that answer, I'm not sure how the comments work
Barry Ort
12,373 PointsBarry Ort
12,373 PointsThanks. I don't know why it still wasn't working the other day, but I started the challenge over tonight and it worked.