Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial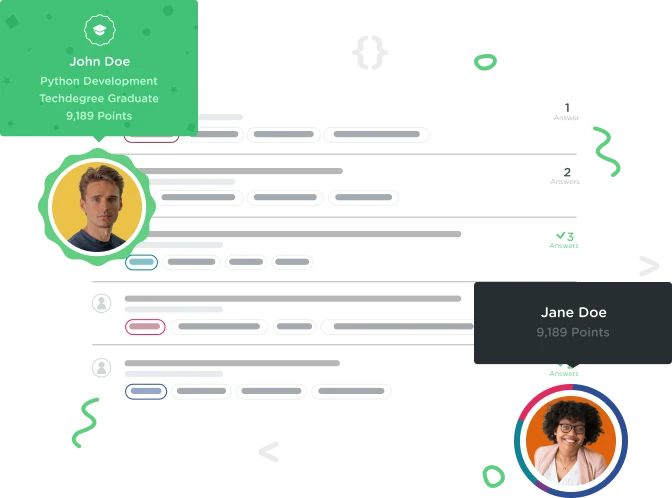

Kenneth Phillips
Courses Plus Student 10,188 PointsWhat is wrong with the boolean method?
I have an app that is a game where if the user gets a question right it will go to the next question. And if it's wrong it will go to an activity where they can submit the score. Everything runs well until I place the boolean method for seeing if the answer is correct. When I do this it marks my variables in my if statement as red and won't recognize them. My onClickListener also stops functioning when I add this in. Before I added this in I had it in an if statement in the onClickListener and the app worked but when I got the question right it would send me to the submit page anyway. Also I haven't added in the score yet so don't worry about that. Here is my code:
public class GameActivity extends AppCompatActivity {
private Game game;
private ImageView gameImageView;
private TextView gameTextView;
private Button submitButton;
private EditText gameEditText;
private Answer gameAnswer;
private int points;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game);
gameImageView = (ImageView) findViewById(R.id.gameImageView);
gameTextView = (TextView) findViewById(R.id.gameTextView);
submitButton = (Button) findViewById(R.id.submitButton);
gameEditText = (EditText) findViewById(R.id.gameSquare);
game = new Game();
loadPage(0);
}
private void loadPage(int pageNumber) {
final String convertedEditText = gameEditText.getText().toString();
final Page page = game.getPage(pageNumber);
final String correctAnswer = page.getAnswer().getCorrectAnswer();
Drawable image = ContextCompat.getDrawable(this, page.getImageId());
gameImageView.setImageDrawable(image);
String pageText = getString(page.getTextId());
gameTextView.setText(pageText);
public boolean verifyAnswer() {
boolean gotItRight = false;
if (convertedEditText == correctAnswer) {
gotItRight = true;
int nextPage = page.getAnswer().getNextPage();
loadPage(nextPage);
} else if (convertedEditText != correctAnswer || convertedEditText == null) {
startSubmit();
}
return gotItRight;
}
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick (View view){
verifyAnswer();
}
});
}
private void startSubmit() {
Intent submitIntent = new Intent(this, SubmitActivity.class);
startActivity(submitIntent);
}
}
3 Answers

Joshua Douce
13,120 PointsHi Kenneth.
At first glance I noticed that you are declaring your public Boolean verifyAnswer() method within your private void loadPage(int pageNumber) method. Then in your verify answer method you are trying to return a Boolean as is it is inside your loadPage method that has a return type of void it will not compile. This may be the answer your looking for give it a try hope it helps.

Kenneth Phillips
Courses Plus Student 10,188 PointsYeah that was the main issue. Also there was a couple of reference problems but I fixed those. Thanks for taking the time to help me. Have a good one.

Joshua Douce
13,120 PointsHappy to help, i find helping helps me solidify what i know and helps me improve

Joshua Douce
13,120 PointsThank you, Caleb, did not even realize.
Caleb Kleveter
Treehouse Moderator 37,862 PointsCaleb Kleveter
Treehouse Moderator 37,862 PointsHi Joshua, I moved your comment to an answer. Keep in mind that posting an answer as a comment prevents it from being upvoted or selected as the best answer.