Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial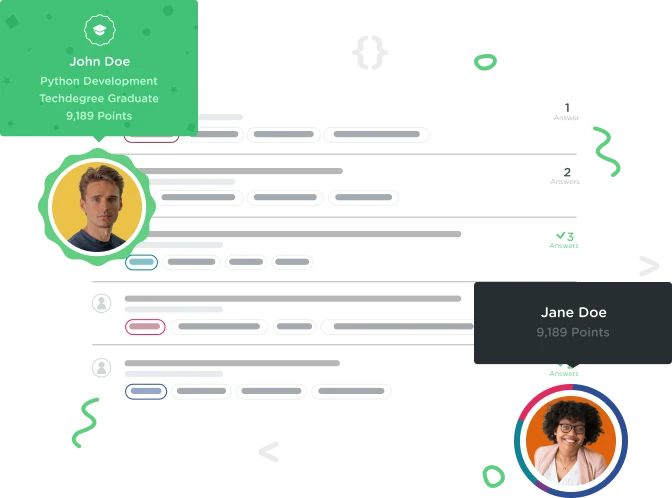

Siddharth Pandey
7,280 PointsWhat is wrong with this animation???
Okay so here's my github file: https://github.com/sid-FBLA/FBLA/tree/master
It may look like a lot at first, but the only three things you need to open to understand this issue are: bookings.html(can be found in HTML folder) bookings.css(can be found in CSS folder, the important part is the bottom, scroll to the styles on the "arrow" class) calc.js(can be found in JS folder, the important part here are lines: 98-end).
Please open my project up on a live server, and click on the book menu(navigation bar).
You will see two select menus, one with the heading "Departure Airport" the other with the heading "Arrival Airport."
Now, you will see that the arrow does not do anything when clicked. However, once you press 'SEARCH FLIGHTS' and then click on an arrow, it works(other than the one on the bottom right, how do I fix this?). Also, you will notice that it only works the first time pressed, not the second time(the class should change from down to up, the arrow should go from a down position to an up position). Also, how can I make it so that if a user clicks on the arrow for it to go down, then when the user clicks on the body, the arrow changes class to go up? Any help is greatly appreciated!
2 Answers
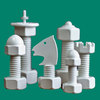
Steven Parker
231,154 PointsYou just need to put the body handler back in, and then add that statement to stop the propagation ("event bubbling") in the handler for the selects, as I suggested in the previous question.
Also, you don't need a separate test for "arrow-up" in the flipper, because by checking first for "arrow-down", if it doesn't have that you know it needs to have that added.
And since you have jQuery loaded, you may as well take advantage of it's more compact syntax:
$("body").click(e => {
$('.arrow').removeClass('arrow-down');
$('.arrow').addClass('arrow-up');
});
Finally, scripts should always be loaded as the very last things in the HTML body area.

Siddharth Pandey
7,280 PointsThanks for all the help! I put the body handler back in and added the e.stopPropogation(); statement. However, now whenever I press the arrow, nothing happens I get a message in the console saying: "Uncaught TypeError: e.stopPropogation is not a function"
Here is my code(I keep the arrow-up class, just because I like it when my classes are specific to the "event" the arrow is in, it just makes it easier for me to read):
function flipper(e) {
let arrow = e.target.previousElementSibling;
if (arrow.classList.contains('arrow-up')) {
arrow.classList.remove('arrow-up');
arrow.classList.add('arrow-down');
e.stopPropogation();
} else if (arrow.classList.contains('arrow-down')) {
arrow.classList.remove('arrow-down');
arrow.classList.add('arrow-up');
e.stopPropogation();
} else {
arrow.classList.remove('arrow-up');
arrow.classList.add('arrow-down');
e.stopPropogation();
}
};
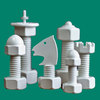
Steven Parker
231,154 PointsIt's just a spelling error ("o" instead of "a"). And you only need to put it in once, before the conditional. Also, a good way to identify functionality without repeated code is with comments:
function flipper(e) {
e.stopPropagation();
let arrow = e.target.previousElementSibling;
if (arrow.classList.contains('arrow-down')) {
arrow.classList.remove('arrow-down');
arrow.classList.add('arrow-up');
} else { // if 'arrow-up' or blank:
arrow.classList.remove('arrow-up');
arrow.classList.add('arrow-down');
}
}
And remember, if you preset "arrow-up" in the HTML you can use "toggle" and no conditional at all.

Siddharth Pandey
7,280 PointsHey Steven! I have a new question regarding something else on my website, out of everyone you have always given me the best answers and been the most helpful. I would really appreciate it if you took a look.
Here is the link to the question: https://teamtreehouse.com/community/why-is-this-not-working-61
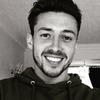
Shaun Kelly
35,560 PointsHi Siddharth,
There are two things I would do here...
In your CSS I would add the following code:
.selected .arrow {
transform: rotate(45deg);
}
And then in your JS i would add the following:
$('#select-1').on("click", function(){
$(this).toggleClass("selected");
});
To snaz it up a bit i would add a transition to the .arrow class.
Hope this helps!

Siddharth Pandey
7,280 PointsThanks for helping, however, this was not my question. I wanted to know how I could make it so that the arrow works(changes class) every time it is pressed. I also want to know how I can make it so that if a user clicks on an arrow for it to go down(changes class to down-arrow), then when the user clicks on the body, the arrow changes class to go up?
Steven Parker
231,154 PointsSteven Parker
231,154 PointsYou forgot the link to the example website //sid-fbla.github.io/FBLA.