Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial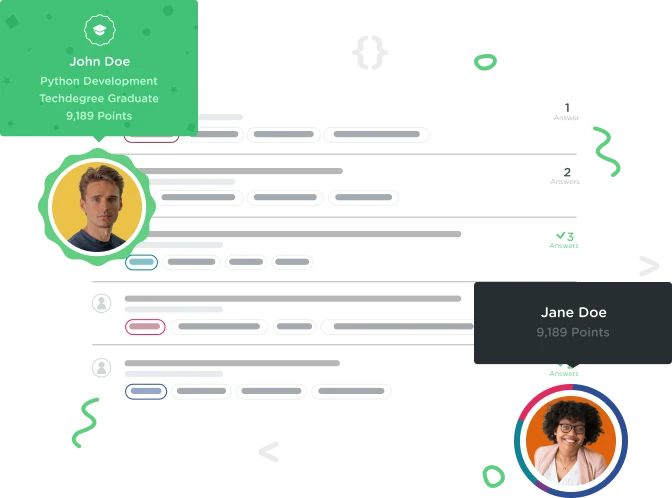

Brok Sorensen
1,578 PointsWhat value is supposed to be in the var echo =?
After your newly created returnValue function, create a new variable named echo. Set the value of echo to be the results from calling the returnValue function. When you call the returnValue function, make sure to pass in any string you'd like for the parameter.
The function will accept the string you pass to it and return it back (using the return statement). Then your echo variable will be set with what is returned, the results.
function returnValue (number){
var echo = returnValue
return number;
}
returnValue("one");
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

andren
28,558 PointsThe echo
variable is meant to be defined outside the function, not inside it. And it should be set equal to calling the function. Like this:
function returnValue(number) {
return number;
}
var echo = returnValue("one");
That will result in echo
being set equal to whatever value the returnValue
function returns.
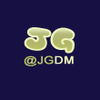
Jonathan Grieve
Treehouse Moderator 91,253 PointsThe value you want to pass in is echo
You want to use the echo outside of the function you create. I'll walk you through each task individually.
To pass the first part, you want to create the basic elements of a function... which is the keyword, the name of the function, a parameter and then the return keyword.
Which gives you the following.
function returnValue (number){
return number;
}
The next thing you want to do is define a new variable, below the function called echo
.
What you'll do is here is call the returnValue
by giving it an argument. The Argument is what the number
paramer is waiting for. It's like a placeholder for a value to be passed into it. And it's assigned to the echo variable so it looks like this
var echo = returnValue("one");
Good luck with the challenge. Hope this clears things up for you :)
Brok Sorensen
1,578 PointsBrok Sorensen
1,578 PointsThat did it! Thank you very much! I was really stumped on that one. Thanks again.