Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial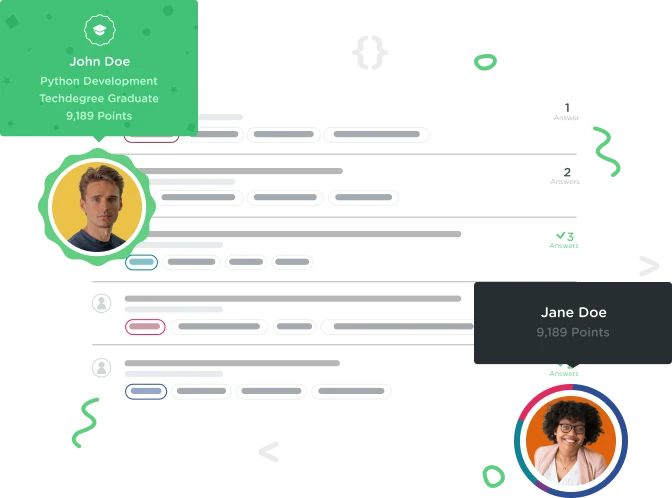

Victor Ngo
8,324 PointsWhat's a more concise way to do this assignment?
SPOILERS BELOW What's a more eloquent way to complete this assignment?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
char mfirstLetter = fieldName.charAt(0);
int indexOfSecondLetter;
// Checks for index of second letter
int letterCount = 0;
int index = 0;
while (letterCount < 2) {
if (Character.isLetter(fieldName.charAt(index))) {
letterCount++;
index++;
}
else {
index++;
}
}
indexOfSecondLetter = index - 1;
char mSecondLetter = fieldName.charAt(indexOfSecondLetter);
//
if (mfirstLetter != 'm' || mSecondLetter != Character.toUpperCase(mSecondLetter)) {
throw new IllegalArgumentException();
}
return fieldName;
}
}
3 Answers

Geoff Parsons
11,679 PointsThe most concise way i would jump to for solving this type of problem would be with regular expressions. They're a bit out of the scope of this course but are quite useful for this type of string validation. If you're not familiar, the gist is you define a pattern using the regular expression syntax and use that to match parts of a string. Conveniently String comes with a matches method that lets us match a string against a regular expression (represented as a string) and get a boolean back with very little extra work on our part.
So we'll need to define a regular expression that matches the pattern. This one should do the trick (though it's far from perfect):
^m[A-Z].*
Let's break down what that does:
- The "^" matches the beginning of the line
- Next we match a lowercase "m"
- Next we match any uppercase letter with "[A-Z]" (note [a-z] would match all lowercase)
- Finally we match any remaining characters with ".*". A period matches any character, and the asterisk means "0 or more of the previous character".
Putting this together we can get a 3-line method that passes the challenge.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if ( !fieldName.matches("^m[A-Z].*") )
throw new IllegalArgumentException();
return fieldName;
}
}
Again, like i said, this is a bit out of the scope for this course but you asked for concise. :)
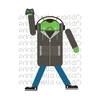
Stephen Bone
12,359 PointsFor those not clued up with Regex like myself (yet!) here's the code I used to pass:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char firstLetter = fieldName.charAt(0);
char secondLetter = fieldName.charAt(1);
if (firstLetter != 'm' || !Character.isUpperCase(secondLetter)) {
throw new IllegalArgumentException();
}
return fieldName;
}
}

Jess Sanders
12,086 Pointsprivate char validateGuess(char letter) {
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required."
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed") }
return letter;
}
The above code is the example to follow.
In pseudocode, we want to check for the following condition, and throw an error: " if the character at index 0 of fieldName does not equal 'm' OR the character at index 1 of fieldName is not upper case"
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("The field name does not match the style");
}
return fieldName;
}
}