Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial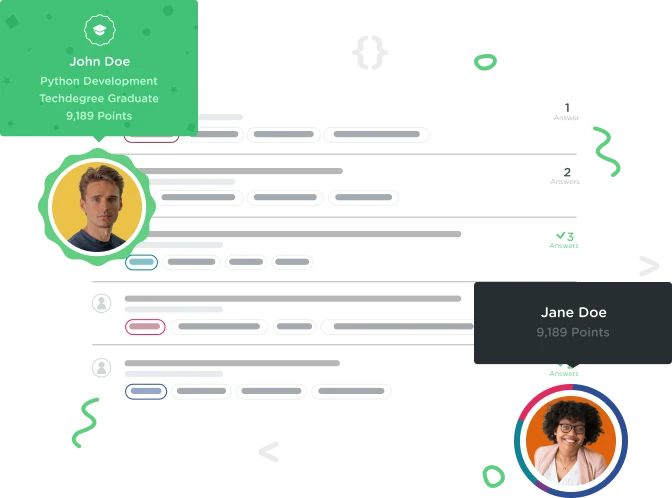

yok558
Courses Plus Student 3,627 PointsWhat's the problem with my IllegalArgumentException guys?
Dont really understand my mistake. Please help.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(20);
}
public void drive(int laps) {
int newAmount = mBarsCount - laps;
if (newAmount < 0) {
throw new IllegalArgumentExeption("Not enough battery remains");
}
mBarsCount = newAmount;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers

Ken Alger
Treehouse TeacherYuri;
Your code looks fine, you are missing a "c" in your Exception. Fix that spelling error and you should be good.
Happy coding and welcome to Treehouse!
Ken

James Simshaw
28,738 PointsYou need to tell Java that your function will throw an exception in the function declaration for example
function someFunction() throws SomeException {
throw new SomeException();
}

Craig Dennis
Treehouse TeacherHi James,
Not yet ;) I used a RuntimeException on purpose, they do not need to be defined. More to come soon!
yok558
Courses Plus Student 3,627 Pointsyok558
Courses Plus Student 3,627 PointsThanks buddy! That spelling..
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherYuri;
Spelling can indeed drive one nuts. Great work on getting the concept though, that is an important step. Remember that during the challenges you can take a look in the Preview section and, generally, get error messages. With the code you posted above you get a cannot find symbol error with the caret pointing to IllegalArgumentExeption.
The cannot find symbol error is Java's way of saying "I don't know what that is!" I wrote a bit about that error in this post. You might take a look just for your reference. As you will discover, learning what the error messages are indicating can help greatly in the debugging process.
Happy coding and pleased that it worked out.
Ken