Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial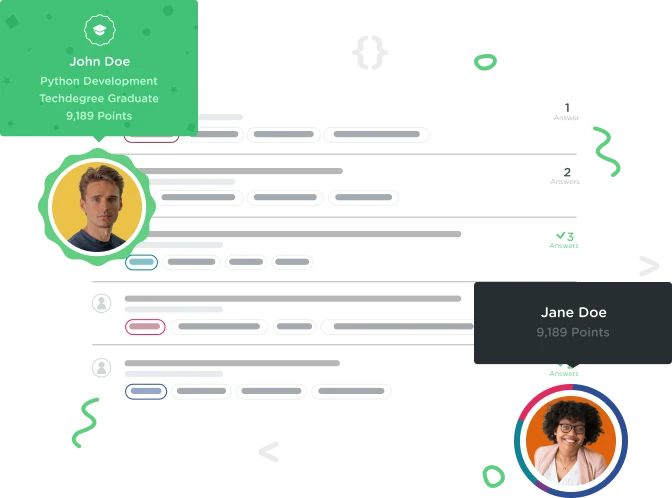
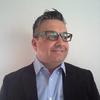
Edgardo Pinto-Escalier Scoffe
2,429 PointsWhat's wrong with my code?
Challenge Task 2 of 2
Call the function and display the results in an alert dialog. You can pass the results of a function directly to the alert() method. For example, to display the results of the Math.random() method in an alert dialog you could type this:
alert( Math.random( ) );
This is my code:
function max(num1, num2) { if (num1 > num2) { return num1; } else{ return num2; } alert( max(num1,num2) ); }
and i get this error:
Bummer! It doesn't look like you used the alert()
method. Try alert( max(2,10) );
function max(num1, num2) {
if (num1 > num2) {
return num1;
}
else{
return num2;
}
alert( max(num1,num2) );
}
8 Answers
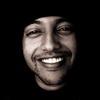
miikis
44,957 PointsSo it looks like you've got it down. It's just you're calling the function inside the block — that's not allowed. It should look like this:
function max(num1, num2) {
if(num1 > num2) {
return num1;
} else {
return num2;
}
}
alert(max(1,2));
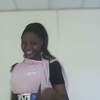
MUZ140639 Elda Mufandarambwa
6,061 PointsPlease help me, l don't know where am getting it all wrong?
function max (a,b){ if(a>b){ return a; }else{ return b; } } alert(max(a,b)); and it says:Oops! It looks like Task 1 is no longer passing.
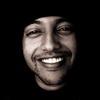
miikis
44,957 PointsHey Elda,
This should really be it's own thread, not a comment on Edgardo's thread... but it's all good.
In any case, your function was constructed correctly, It's the alert call that's causing the error — the arguments cannot be letters, they have to be numbers, such as 1 and 2 (a cannot be less-than b, at least not in the way you're thinking). Below is the correct code:
function max(a, b) {
// "a" and "b" are parameters (think placeholders) for this function
if(a > b) {
return a;
} else {
return b;
}
}
//When you call the function, you need to replace those "placeholders" with actual numbers, in this case.
alert(max(1,2));
//The returned value of the alert call should be the number 2, since 1 is not greater-than 2.
Hope that helps.
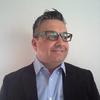
Edgardo Pinto-Escalier Scoffe
2,429 PointsOhhh yes thanks a lot for pointing out that, of course i was inside the same block ;)
Thanks for your help, now it works!
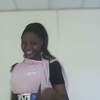
MUZ140639 Elda Mufandarambwa
6,061 PointsHey Mikis Thanks so much for highlighting that. Had tried numbers and it did worked but was still wondering what was wrong with letters. Thanks for the reminder once again.
Elda
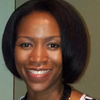
Natasha Johnson
12,285 PointsMikis, You are correct! Thanks for the explanations also.
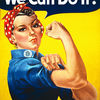
Siyuan Welch
3,025 Pointsthanks , Mikis! You also help me a lot!

Rafal Kita
5,496 PointsI solved it this way
function max(x, y) {
var bigger = Math.max(x, y);
return bigger;
}
alert(Math.max(15, 150));
and i took it from there [http://www.w3schools.com/jsref/jsref_max.asp ] Yeah this wasn't easy for me either. Mikis explained it very well, thanks
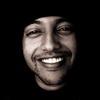
miikis
44,957 PointsHey Rafal,
So, yeah, you could use Math.max() to solve this Challenge. And when you're building software in the real world you would, indeed, use built-in functions like Math.max() whenever applicable. But, the point of this particular Challenge was to teach you how to build your own functions. And since functions are such an integral part of JavaScript programming, I would suggest you now try to build some functions of your own to make sure that you've truly grokked the concept. Good luck :)

claudius mainja
9,661 Pointsthanks for explanation but why are we replacing the letters a& b with the numbers there
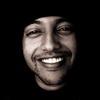
miikis
44,957 PointsBecause that's the whole point of functions: to perform actions based on inputs and — optionally — return some output. a & b were placeholders for inputs; 1 & 2 are the inputs. The function called max figures out which one is bigger, a or b. Then it returns the result of that comparison as its output — it returns 2.

claudius mainja
9,661 PointsThank you Mikis