Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial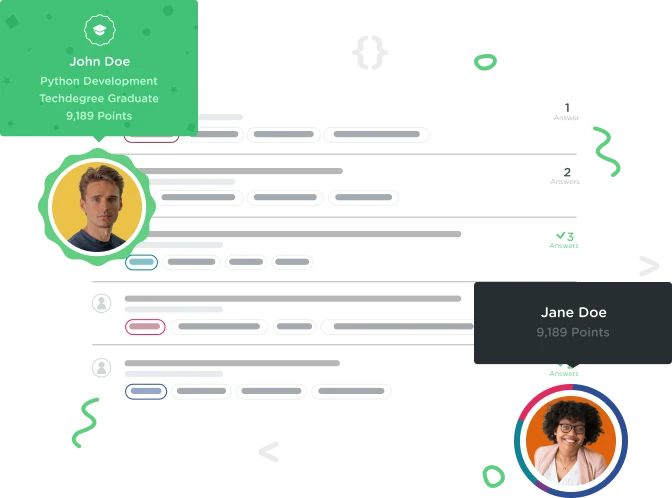
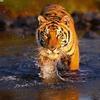
Konrad Pilch
2,435 PointsWhats wrong with this code?
What is wrong with this code? it makes no effect :
FILE NAME: connect.inc.php
CODE:
<?php
$mysqli_host = 'localhost';
$mysqli_user = 'root';
$mysqli_pass = 'root';
$mysqli_db = 'a_database';
if (!mysqli_connect($mysqli_host, $mysqli_user, $mysqli_pass) || !mysqli_select_db($mysqli_db)) {
die(mysqli_error());
}
?>
Index:
<?php
require 'connect.inc.php';
?>
3 Answers
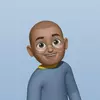
Ash Matadeen
Courses Plus Student 7,185 PointsOk, so a couple of issues here.
The mysqli_select_db() function expects 2 arguments, in addition to the database name, you need to pass it the database link - this is the object returned by mysql_connect().
The mysqli_error() function also expects the object returned by mysql_select_db() as argument.
Try this:
<?php
error_reporting(E_ALL); // reports all errors to make it easier to debug
$connection = mysqli_connect($mysqli_host, $mysqli_user, $mysqli_pass);
if (!$connection) {
die("Could not connect to the database server");
} elseif (!mysqli_select_db($connection, $mysqli_db)) {
echo "Could not select the database<br />";
die(mysqli_error($connection));
} else {
echo "Connection successful";
}
?>
If you then change the database name, or login credentials, you should see the relevant error.
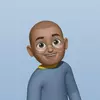
Ash Matadeen
Courses Plus Student 7,185 PointsKonrad Pilch The key difference is in the arguments that you pass through to mysqli_select_db() and mysqli_error().
<?php
mysqli_select_db($mysqli_db); // will not work as it's missing the $connection argument
mysqli_select_db($connection, $mysqli_db); // will work
mysqli_error(); // will not work as it's missing the $connection argument
mysqli_error($connection); // will work
?>
It's worth having a look at the documentation for those two functions: mysqli_select_db() documentation mysqli_error() documentation
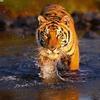
Konrad Pilch
2,435 PointsThank you! :)
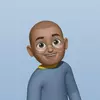
Ash Matadeen
Courses Plus Student 7,185 PointsIf you don't see any errors when loading this script, it means that your connection to the database was successful.
<?php
if (!mysqli_connect($mysqli_host, $mysqli_user, $mysqli_pass) || !mysqli_select_db($mysqli_db)) {
die(mysqli_error());
}
?>
This bit of code stops the script and displays any errors BUT only if it has either failed to connect to the mysql server or failed to select the database.
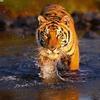
Konrad Pilch
2,435 Pointsyes but then i did make and error for the purpose of checking and i had no errors shown .
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsThank you ! It worked. But why my code didnt work? whats the difference?