Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial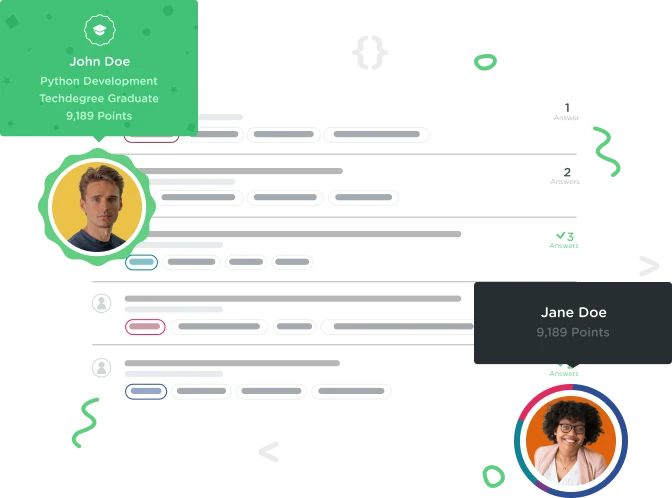

eddie chen
3,790 PointsWhen adding if condition to the code, I got confused...Can anyone help a little bit?
The questions are below.
Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers.
HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
function max (10,20){
if (10<20){
return 20;
}
}
3 Answers
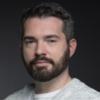
Cory Harkins
16,500 PointsNo worries, you don't always need an else condition though. :)
Just ran the challenge with a pass
function max(a,b) {
if (a < b) {
return b;
}
}
alert(max(5,7));
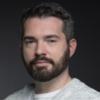
Cory Harkins
16,500 PointsWhen you assign integers as arguments, you are "hard coding" that argument.
You want to get away from that black/white programming concept and think of fluidity and re-usable code.
function max(a,b) {
if (a < b) {
return(a);
}
}
In this example, I have left the arguments to be whatever the input from user is. As (a) becomes a variable, and the data that get's "passed" into that argument is stored by (a). The same is with (b).
The return method is your choice. There are many different statements to execute from an if/else statement.
The fluidity comes from the function call...
max(3,8);
What if you want to change the value returned from max throughout your program as a dynamic integer?
if you call YOUR function max(10,20); the result will always be the same, but when we assign arguments to store the data, the process becomes very fluid.

eddie chen
3,790 Pointsthanks a lot man, that's very specific!

eddie chen
3,790 PointsHey, man, I follow the code you provided, but it says there is a syntax error. any idea which part?
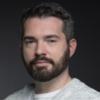
Cory Harkins
16,500 Pointsfunction max (a,b) {
if (a < b) {
return b;
}
}
The challenge asks that you return the larger of the two, and no parenthesis around the value result of your return.
:)

eddie chen
3,790 PointsAnother student just told me that I was missing an " else" condition, and once I added it, it worked. Again, thanks for helping.