Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial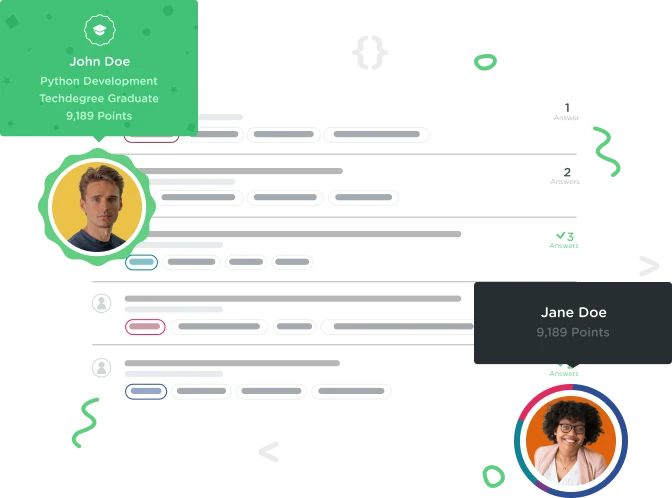

Tobi Ogunnaike
2,242 PointsWhen calling a function that has an argument, why is it that sometimes you need to specify the parameter..
When calling a function that has an argument, why is it that sometimes you need to specify the parameter and sometimes you don't
See these two examples from code challenges I've solved-
function max(larger, smaller) {
if(parseInt(larger) > parseInt(smaller)){
return parseInt(larger);}
else {return parseInt(smaller)}
}
alert (max());
In the above case , the max() function is called without me needing to write
alert( max(larger, smaller)
However in a prior code challenge
function returnValue (drink){
return drink}
returnValue('coffee');
var echo = returnValue('coffee');
Or do I have to ALWAYS have to specify a parameter when calling a function because the browser would not otherwise know what the argument is?
I'm confused and I appreciate any help thanks!
1 Answer
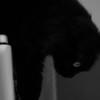
moonshine
8,449 PointsJavaScript is forgiving because leaving off arguments in languages like C++ would cause errors. On a side note, the parameters you give a function refer to the indexes of an array called arguments.
function foo() {
console.log(arguments[0]);
}
console.log(foo(2)); /* Prints 2 */
function boo(var_a) {
console.log(var_a === arguments[0]);
}
boo("text"); /* Prints true */
Arguments that are not passed to a function will simply be undefined, and as long as your function doesn't try to do something like undefined.charAt(2) it will run without errors.
If parseInt(undefined) === NaN then calling max would look like:
function max(undefined, undefined) {
if (NaN > NaN){
return NaN;
} else {
return NaN;
}
}
alert (max()); /* Alerts NaN. */
if we replace the parameters with their actual values.
mickey Odunikan
4,983 Pointsmickey Odunikan
4,983 PointsNot sure if you put on the entire code but when calling a function it is only necessary to add in parameter if the function needs that information. The function may need user input or other information from previously written code in order to work the way you want.
hope that helps