Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial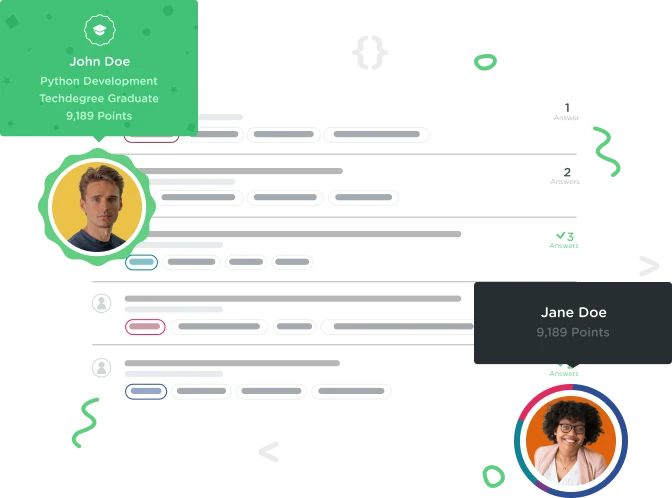
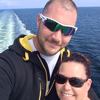
Shawn Wilson
iOS Development Techdegree Student 7,049 PointsWhen testing in my app to see if the form will store to MYSQL DB it tells me a field cant be blank when it is not.
Hey guys im working on a personal project here and when i fill out my form in the actual application on localhost:3000, it tells me the feilds cant be blank.. the thing is they are not blank..
Here is my users_controller:
class UsersController < ApplicationController
before_action :set_user, only: [:show, :edit, :update, :destroy]
def index
@user = User.all
end
#GET /users/new
def new
@user = User.new
end
#GET /users/1/edit
def edit
end
# POST /users
# POST /users.json
def create
@user = User.new
respond_to do |format|
if @user.save
format.html { redirect_to @user, notice: 'Employee was successfully created.' }
format.json { render action: 'show', status: :created, location: @user }
else
format.html { render action: 'new'}
format.json { render json: @user.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /users/1
# PATCH/PUT /users/1.json
def update
respond_to do |format|
if @user.update(user_params)
format.html { redirect_to @user, notice: 'Employee was successfully updated.'}
format.json { head :no_content }
else
format.html { render action: 'edit'}
format.json { render json: @user.errors, status: :unprocessable_entity }
end
end
end
# DELETE /users/1
# DELETE /users/1.json
def destroy
@user.destroy
respond_to do |format|
format.html { redirect_to users_url }
format.json { head :no_content }
end
end
private
# uses callbacks to share common setup / constraints between actions
def set_user
@user = User.find(params[:id])
end
def user_params
params.require(:user).permit(:first_name, :middle_name, :last_name, :badge_number, :telephone, :email, :password_digest)
end
end
Here is my _form.html.erb
<%= form_for(@user) do |f| %>
<% if @user.errors.any? %>
<div id="error_explination">
<h2><%= pluralize(@user.errors.count, 'error') %> prohibited this employee from being saved:</h2>
<ul>
<% @user.errors.full_messages.each do |msg| %>
<li><%= msg %></li>
<% end %>
</ul>
</div>
<% end %>
<div class="name_field">
First Name: <%= f.text_field :first_name %>
Middle Name: <%= f.text_field :middle_name %>
Last Name: <%= f.text_field :last_name %>
</div>
<div class="employee_info">
Badge Number: <%= f.text_field :badge_number %>
Telephone Number: <%= f.text_field :telephone %>
Email Address: <%= f.text_field :email %>
</div>
<div class="employee_password">
Password: <%= f.password_field :password %>
Confirm Password: <%= f.password_field:password_confirmation %>
</div>
<div class="actions">
<%= f.submit %>
</div>
<% end %>
here is my user model:
class User < ActiveRecord::Base
has_secure_password
validates :email, presence: true
validates :badge_number, presence: true
before_save :downcase_email
def downcase_email
self.email = email.downcase
end
end
here is my new.html.erb:
<h1>Create New Employee</h1>
<%= render 'form' %>
<%= link_to 'Back', users_path %>
it will sometime generate a blank record within the Database, and then will accept the entries with an update user entry.. not sure what is going on and cant seem to find much help resolving it...
2 Answers
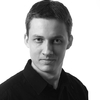
Maciej Czuchnowski
36,441 PointsYou have to add user_params to your create action in the controller:
@user = User.new(user_params)
Right now the code does not allow any parameters to be saved in the database when creating new records. This should help you understand the concept: http://www.lynda.com/Ruby-Rails-tutorials/Mass-assignment-strong-parameters/139989/159116-4.html
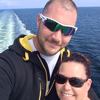
Shawn Wilson
iOS Development Techdegree Student 7,049 PointsSo i think i should just get it over with and hire you lol..! thanks for the help here! i knew it looked naked but couldnt put my finger on it! thanks Maciej! as always you've been a huge help!
Shawn Wilson
iOS Development Techdegree Student 7,049 PointsShawn Wilson
iOS Development Techdegree Student 7,049 PointsWhen i run it with Rails Server open this is what it returns in the log:
Started POST "/users" for ::1 at 2015-04-13 00:01:12 -0600 Processing by UsersController#create as HTML Parameters: {"utf8"=>"✓", "authenticity_token"=>"+ggWc20qIZW0kYKKwGNUXKsSRRqz7WWB64leKldplE76NbO7qjQO5/fFQ5qdkGhj7Hk778yB6ozIwdEaMdanlQ==", "user"=>{"first_name"=>"John", "middle_name"=>"Deer", "last_name"=>"Doe", "badge_number"=>"4116", "telephone"=>"111-222-3333", "email"=>"test1@youremail.com", "password"=>"[FILTERED]", "password_confirmation"=>"[FILTERED]"}, "commit"=>"Create User"} (0.1ms) BEGIN (0.1ms) ROLLBACK Rendered users/_form.html.erb (8.9ms) Rendered users/new.html.erb within layouts/application (9.9ms) Completed 200 OK in 48ms (Views: 43.0ms | ActiveRecord: 0.2ms)