Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial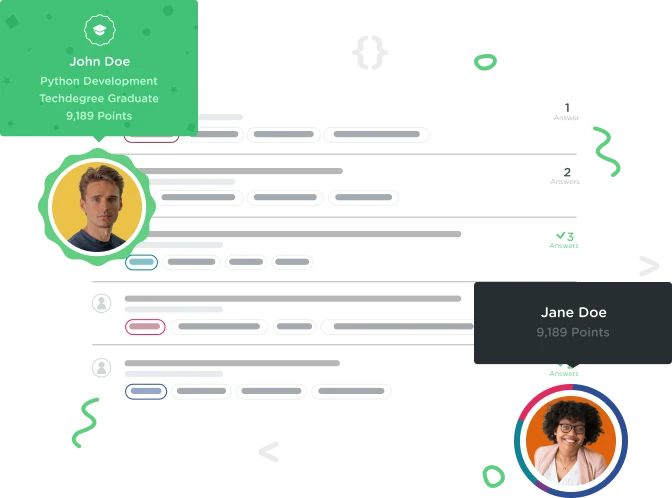

Kylie Nonemaker
1,190 PointsWhen to use arguments in my method?
Want to make sure I understand when I would add arguments to a method. I'm on the iter lesson and sometimes we add arguments to methods and others do not. From my understanding, we would add an argument as we did below so that when we create an instance we set that argument? Whereas, if we don't use the argument we can't pass in that attribute when creating the instance. Is this correct?
class Dealership:
def __init__(self):
self.cars = []
def __iter__(self):
yield from self.cars
def add_car(self, car):
self.car.append(car)
2 Answers
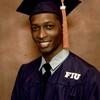
Dane Parchment
Treehouse Moderator 11,077 PointsTLDR: You use arguments whenever you want to parametrize your methods, which basically means making your methods output be affected by arguments provided by the user. (This isn't **exactly* what it means, but in this case it's close enough)*
So basically you want to use arguments whenever you want the output of your method to be affected by some sort of input. Let's say for example that you are creating a method that adds two numbers together.
def add():
return 1 + 2
This is perfectly valid code, but whenever you use this, it will always just add 1 and 2. This is kind of pointless right? So let's change that to use some variables, that way we can change what the numbers are.
a = 1
b = 2
def add():
return a + b
Okay, this is a bit better, we are using variables now, so we can change the numbers to whatever we want rather than just having them hard coded. But now we've run into another issue. Whoever is using your add
method, may not have access to those variables (for example they may be importing your add method and using it in their code), or if they do have access those variables can change throughout the life of the program before the add method is called.
So to solve this, we will make the method use arguments, that way when the method is called the user can specify exactly what they want added together. Let's do that by extracting the variables into arguments.
def add(a, b):
return a + b
Great! We've done it! Now our method is using arguments!
But! When do we not use arguments? Basically if we know we don't need any sort of input to change the output of our method. To keep it simple, lets say we have a method that simply prints hello;
def say_hello():
print("Hello.")
In this case, we don't need any sort of input to change what the output of this method is, because we will always just be saying hello!
Hopefully that clears things up for you!
P.S. One more thing to go over! Class methods are a unique exception to the rule above. As whenever we are creating a method in a class, they will implicitly always be using an argument called self
, this is something python requires in order for the class method to properly interact with the class and its internal state. For example:
class Person:
def __init__(self, name):
self.name = name
def say_hello(self):
print(f"{self.name} says hello!")
def walk(self):
print("Walks in a general direction")
In this case you can see that we will need the self
argument whenever we need to access the internal state of our class (in this case, self.name
). But you also notice that we require this self argument even when we don't need it like in the walk
method. This is because a class method has the self
argument as an implicit parameter. Which means that the method is implied and doesn't need to explicitly set in the method call. For example:
class Person:
def __init__(self, name):
self.name = name
def say_hello(self):
print(f"{self.name} says hello!")
def walk(self):
print("Walks in a general direction")
me = Person("Dane")
person.walk() # Walks in a general direction
See we don't need to actually place any argument in the call because the self is implied, the class itself will fill that in. However, if we were to not add the self parameter when defining the method in the class, we would get an error.
class Person:
def __init__(self, name):
self.name = name
def say_hello(self):
print(f"{self.name} says hello!")
def walk(): # notice that we aren't using the self parameter?
print("Walks in a general direction")
me = Person("Dane")
person.walk() # Would give an argument count error
Again hopefully that clears things up for you, if you need more help or clarification let me know.
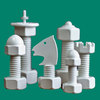
Steven Parker
241,771 PointsIf a property that an object requires will always be known when it is created, it makes sense to have an argument to pass to set it up at that time.
But if an object can exist without any initial attributes (they might be added later), no arguments are needed when creating it.
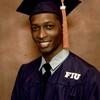
Dane Parchment
Treehouse Moderator 11,077 PointsHaha, you beat me to it in less words, I may have gone overboard with my answer.

Anders Beutler
Courses Plus Student 606 PointsMay have gone overboard on the answer, Dane, but it helped explain a lot for me and I'm not on that subject yet!
Steven Parker
241,771 PointsSteven Parker
241,771 PointsYou might want to fix the unbalanced parentheses in the examples.
Dane Parchment
Treehouse Moderator 11,077 PointsDane Parchment
Treehouse Moderator 11,077 PointsGood catch