Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial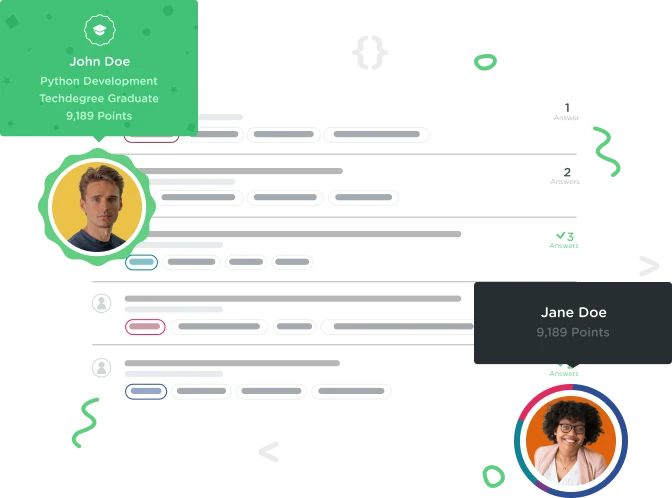

Abe Daniels
Courses Plus Student 2,781 PointsWhere do I start with this?
"So at your new Java job, you've written a brand new Shopping Cart system for the website. After some developers have used the objects you created for a while, they ask you to make it easier to add things to the cart.
Check out more instructions in Example.java. Once you implement their request, check your work, and you will pass the challenge."
I'm having troubles understanding what is being asked and what is going on here. Can someone give me a low-down?
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}

Abe Daniels
Courses Plus Student 2,781 Pointsupdated with question.
2 Answers

Daniel Babbev
10,354 PointsCraig has given you a real life example. When you work for a company your fellow colleagues will leave comments in the code. Cuz, you know, there is no code challenge engine in companies. You should complete all the tasks he has given you and then submit the code by clicking the next button. If you have questions about the code challenge itself please ask, I'll be happy to answer. Cheers, have fun coding ;)

Jonathan Seed
1,223 PointsI have not done this exercise myself, but I believe I know what is being asked.
So right now, if the user wants to add an item to the cart, they must call the addItem method, passing the product and an quantity as arguments.
So currently, if you wanted to add just one of the product, calling the method would look like .addItem(Product, 1);
You are being asked to create a new overloaded method that allows the user to add a single product without specifying a quantity.
The desired functionality is demonstrated with the following code:
cart.addItem(dispenser);
To make this work, you will need to create a new addItem method in the shopping cart class that accepts only one parameter, the product!

Daniel Babbev
10,354 PointsYou have to define second constructor in the Cart object that takes one parameter only. In java we can have multiple constructors. Here is an example:
public class Cart{
private Item mItem;
private int mQuantity;
//Some fields here
public Cart(Item item, int quantity){ // This is the constructor #1
mItem = item;
mQuantity = quantity;
}
public Cart(Item item){ //This is constructor #2 you need to provide in order to complete the challenge
mItem = item;
mQuantity = 1; //This time we set quantity to be 1 by default
}
}
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsCan you post the question to this challenge?