Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial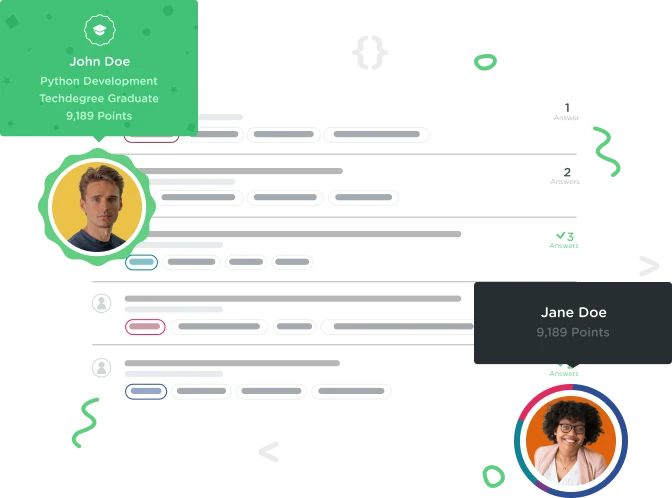

CheVon Bell
491 PointsWhere is the while statement supposed to go?
I'm not sure where I'm supposed to put the while statement. I've tried putting it various different places, but I keep getting an error. Is there something I'm doing wrong?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
1 Answer
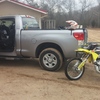
Ryan Ruscett
23,309 PointsHey, let's start from the beginning.
QUESTION Okay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method. Let's make it so it will only charge until the battery reports being fully charged. Let's use the ! symbol and a while loop. Inside the loop increment mBarsCount.
Let's break this up.
- Use isFullyCharged to change our implementation of the charge method.
- Let's use the ! symbol which we know ! reverses the value of a boolean.
- We need a while loop to increment the mBarsCount
Ok so I know by number 1, I need the while loop to go inside the charge method. Since that is the one I am changing the implementation of.
I know by number 2 that I need to use the ! symbol. If I look at the helper method, which helps me accomplish this. I see right away that it's returning me a value.
return mBarsCount == MAX_ENERGY_BARS;
Wait wait wait!! Ok So the double equals or == means it's an equality check. The double equals holds a lot of power in comparing objects but I will spare you those details. What it basically means here is that I am going to get a true or false value back. Either mBarsCount is equal to MAX_ENERGY_BARS and I will get a true or it's not and I will get a false.
This plays right into !, which means the opposite. So if I call my hellper method, in a while loop as the condition. I can do it like this.
public void charge() {
while(!isFullyCharged()) {
Ok so isFullyCharged tells me if my battery is fully charged by returning a true or false value. So if I use the ! which says the opposite. Basically while isFullyCharged is NOT true, do something.
That something has to be taking mBarsCount and adding to it. I can do that one of two ways.
mBarsCount++ or mBarsCount = mBarsCount + 1
The mBarsCount++ is the preferred way.
Now, let's bring this all together.
- In the Charge method, I will call isFullyCharged. Which returns a True or False value. I want this to run until that method returns True. So I will use the ! the opposite of True. So while !isFullyCharged() meaning as long as the method returns something other that True, which is desired, do something. That something in incrementing mBarsCount. Add one to it, and then run the mthod again. If the method returns false, run the method over and over until it returns true,
public void charge() {
while(!isFullyCharged()) {
mBarsCount++;
}
mBarsCount = MAX_ENERGY_BARS;
}
It's important to note that mBarsCount is a member variable or class variable. Meaning that when I do mBarsCount++ it's adding 1 to it, when I call isFullyCharged, it's checking the member variable or class variable to see what it is. If it's not 8 than I keep going. Both of these methods are using the same variable. that is why it's a member or class variable. So each method can access it without an issue with scope.
Does this help? If not let me know and I can try to approach it a different way.
CheVon Bell
491 PointsCheVon Bell
491 PointsI implemented your suggestions and it worked! Thanks a lot!