Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial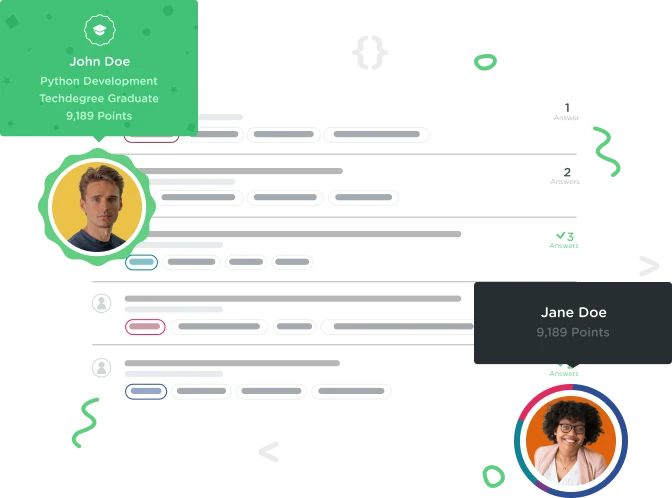

Sarvesh Tandon
1,142 PointsWhy am I getting a null drawable? Weird error. Please Help!! :~(
05-27 23:40:57.218 9172-9210/com.sarveshtandon.www.stormy I/MainActivity: 11:11AM
05-27 23:40:57.225 9172-9172/com.sarveshtandon.www.stormy D/AndroidRuntime: Shutting down VM
05-27 23:40:57.226 9172-9172/com.sarveshtandon.www.stormy E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.sarveshtandon.www.stormy, PID: 9172
java.lang.NullPointerException: Attempt to invoke virtual method 'void
android.widget.ImageView.setImageDrawable(android.graphics.drawable.Drawable)' on a null object reference
at com.sarveshtandon.www.stormy.MainActivity$1$1.run(MainActivity.java:83)
at android.os.Handler.handleCallback(Handler.java:769)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6540)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
package com.sarveshtandon.www.stormy;
import android.app.Activity; import android.databinding.DataBindingUtil; import android.graphics.Bitmap; import android.graphics.drawable.Drawable; import android.nfc.Tag; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.text.method.LinkMovementMethod; import android.util.Log; import android.widget.ImageView; import android.widget.TextView;
import com.sarveshtandon.www.stormy.databinding.ActivityMainBinding;
import org.json.JSONException; import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
private static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather currentWeather;
ImageView icon;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final ActivityMainBinding binding = DataBindingUtil.setContentView(MainActivity.this, R.layout.activity_main);
TextView darkSkyView = findViewById(R.id.darkSkyView);
darkSkyView.setMovementMethod(LinkMovementMethod.getInstance());
String apiKey = "e9267719c11970d4e600f3cd50f7e94c";
double latitude = 37.8267;
double longtude = -122.4233;
String forecastURL= "https://api.darksky.net/forecast/" + apiKey + "/" + latitude + "," + longtude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastURL).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String JSONData = response.body().string();
Log.v(TAG, JSONData);
if(response.isSuccessful()){
currentWeather = getCurrentDetails(JSONData);
final CurrentWeather displayWeather = new CurrentWeather(
currentWeather.getLocationLabel(),
currentWeather.getIcon(),
currentWeather.getTime(),
currentWeather.getTemperature(),
currentWeather.getHumidity(),
currentWeather.getPrecipChance(),
currentWeather.getSummary()
);
Log.i(TAG,displayWeather.getFormattedTime("America/Los_Angeles"));
Log.i(TAG,String.valueOf(displayWeather.getIcon())+"hey");
Log.i(TAG, String.valueOf(R.drawable.clear_day));
Drawable drawable = getResources().getDrawable(currentWeather.getIconId());
Log.i(TAG,displayWeather.getFormattedTime("America/Los_Angeles"));
binding.setWeather(displayWeather);
runOnUiThread(new Runnable() {
@Override
public void run() {
Drawable drawable = getResources().getDrawable(currentWeather.getIconId());
icon.setImageDrawable(drawable);
}
});
}
else{
alertUserAboutTheError();
}
} catch (IOException e) {
Log.e(TAG, "IOException Caught:" ,e);
} catch (JSONException e){
Log.e(TAG, "JSONException caught:",e);
}
}
});
}
private CurrentWeather getCurrentDetails(String data) throws JSONException {
JSONObject forecast = new JSONObject(data);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON:"+timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setLocationLabel("Alcataraz Island, CA");
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
Log.i(TAG, currentWeather.getFormattedTime(timezone));
return currentWeather;
}
private void alertUserAboutTheError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
1 Answer

Seth Kroger
56,416 PointsIt looks like you declared the icon variable, but you forgot to initialize it through findViewById() in onCreate().

Sarvesh Tandon
1,142 PointsIt works now. Thanks!!
Sarvesh Tandon
1,142 PointsSarvesh Tandon
1,142 PointsCheck this out! even when I am inputing an ID which exists it is giving me the following error. PS I have already tried putting a try catch for a NULLexception