Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial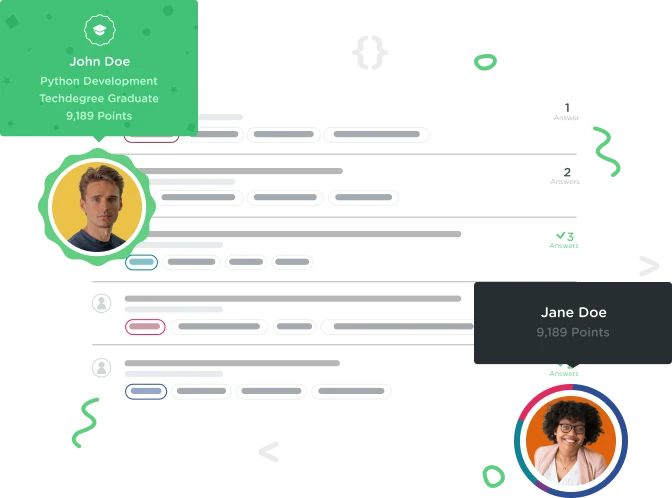
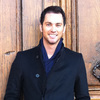
M Fen
9,858 PointsWhy do I get "=> nil" after creating an attr_reader : transactions method, reloading the file, making deposits etc?
created the attr_reader : transactions on the top of my file per the video but why does it return "nil" after I save the file, reload in irb, making some deposits and withdraws and then call on bank_account.transactions in IRB?
Is it because it was never defined in the initialize method?
class BankAccount
#Attribution
attr_reader :balance
attr_reader :transactions
# Class Methods
class << self
def create_for(first_name, last_name)
@accounts ||= []
@accounts << BankAccount.new(first_name, last_name)
end
def find_for(first_name, last_name)
@accounts.find{|account| account.full_name =="#{first_name} #{last_name}"}
end
end
#Instance Methods
def initialize(first_name, last_name)
@balance = 0
@first_name = first_name
@last_name = last_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def deposit(amount)
@balance += amount
end
def withdraw(amount)
@balance -= amount
end
end
2 Answers
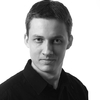
Maciej Czuchnowski
36,441 PointsI don't see any line which would instantiate or modify the @transactions variable in any way, so you can't expect it to contain anything. Basically you have a method for reading the contents of @transactions variable that is always empty (nil) because you never did anything to any variable called @transactions at any point in the code.
Look at @balance: it starts off as 0 in the initialize, so whenever you create a new account, you can read its balance and you will get at least 0, and then you have two methods that modify that variable (deposit and withdraw, and they do something to that variable).
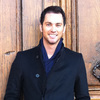
M Fen
9,858 PointsThat makes sense. I figured it was because it was never added to the init method.
Thanks a mill for the working example too.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsIn order to have this working in the most basic version (counting the number of transactions made in the account), you would have to add this to your initialize method:
@transactions = 0
and this to both your deposit and withdraw methods:
@transactions += 1
This will add 1 to transactions of that account anytime you deposit or withdraw money from it.