Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial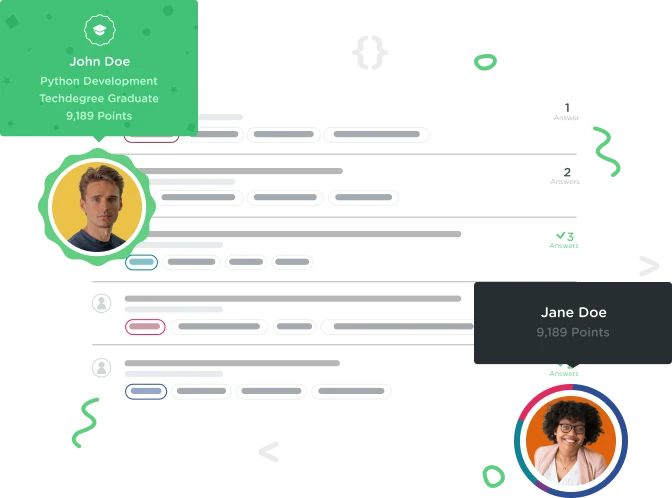
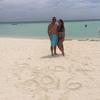
Javier Gandia
Full Stack JavaScript Techdegree Student 9,153 PointsWhy do I keep getting error...
HTML: <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Random Quotes</title> <link href='https://fonts.googleapis.com/css?family=Playfair+Display:400,400italic,700,700italic' rel='stylesheet' type='text/css'> <link rel="stylesheet" href="css/normalize.css"> <link rel="stylesheet" href="css/styles.css"> </head> <body> <div class="container"> <div id="quote-box"> <p class="quote"></p> <p class="source"> <span class="citation"></span><span class="year"></span></p> </div> <button id="loadQuote">Show another quote</button> </div> <script src="js/script.js"></script> </body> </html>
JavaScript: //quotes array. quote, source, citation, year. const quotes = [ {quote:"This election is a total sham and a travesty. We are not a democracy!", source:"@realDonaldTrump", citation:"Twitter", year:"6 Nov 2012"}, {quote:"Obama is, without question, the WORST EVER president. I predict he will now do something really bad and totally stupid to show manhood!", source:"@realDonaldTrump", citation:"Twitter", year:"5 Jun 2014"}, {quote:"If Obama resigns from office NOW, thereby doing a great service to the country—I will give him free lifetime golf at any one of my courses!", source:"@realDonaldTrump", citation:"Twitter", year:"10 Sep 2014"}, {quote:"An 'extremely credible source' has called my office and told me that @BarackObama's birth certificate is a fraud.", source:"@realDonaldTrump", citation:"Twitter", year:"6 Aug 2012"}, {quote:"Robert Pattinson should not take back Kristen Stewart. She cheated on him like a dog & will do it again--just watch. He can do much better!", source:"@realDonaldTrump", citation:"Twitter", year:"17 Oct 2012"}, {quote:"Hillary Clinton has announced that she is letting her husband out to campaign but HE'S DEMONSTRATED A PENCHANT FOR SEXISM, so inappropriate!", source:"@realDonaldTrump", citation:"Twitter", year:"26 Dec 2015"}, {quote:"The election is absolutely being rigged by the dishonest and distorted media pushing Crooked Hillary - but also at many polling places - SAD", source:"@realDonaldTrump", citation:"Twitter", year: "16 Oct 2016"}, {quote:"I refuse to call Megyn Kelly a bimbo, because that would not be politically correct. Instead I will only call her a lightweight reporter!", source:"@realDonaldTrump", citation:"Twitter", year:"27 Jan 2016"}, {quote:"I am going to repeal and replace ObamaCare. We will have MUCH less expensive and MUCH better healthcare. With Hillary, costs will triple!", source:"@realDonaldTrump", citation:"Twitter", year:"2 Nov 2016"}, {quote:"The concept of global warming was created by and for the Chinese in order to make U.S. manufacturing non-competitive.", source:"@realDonaldTrump", citation:"Twitter", year:"6 Nov 2012"}, {quote:"It's freezing and snowing in New York--we need global warming!", source:"@realDonaldTrump", citation:"Twitter", year:"7 Nov 2012"}, {quote:"Sorry losers and haters, but my I.Q. is one of the highest -and you all know it! Please don't feel so stupid or insecure,it's not your fault", source:"@realDonaldTrump", citation:"Twitter", year:"8 May 2013"}, ];
//function to randomize array of quotes. const getRandomQuote = () => { let random = Math.floor(Math.random() * quotes.length); return quotes[random]; };
//function to add text to a specific class on the html. const printQuote = () => { let randomQuote = getRandomQuote(); document.getElementsByClassName("quote")[0].innerHTML = randomQuote.quote; document.getElementsByClassName("source")[0].innerHTML = randomQuote.source; document.getElementsByClassName("citation")[0].innerHTML = randomQuote.citation; document.getElementsByClassName("year")[0].innerHTML = randomQuote.year; };
//console.log(printQuote()); //for loop to add a different quote on load for (let i = 0; i < quotes.length; i++) { printQuote(); };
// event listener to respond to "Show another quote" button clicks // when user clicks anywhere on the button, the "printQuote" function is called document.getElementById('loadQuote').addEventListener("click", () => { printQuote();
});
This is the complete code. Works fine expect that it says that the citation is undefined.. If i remove: document.getElementsByClassName("source")[0].innerHTML = randomQuote.source;
gives no error and all text is added on page like normal, except of course for the "source array"
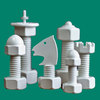
Steven Parker
241,958 PointsThe instructions for code formatting are in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
You could also make a snapshot of your workspace and post the link to it here.
1 Answer
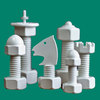
Steven Parker
241,958 PointsYour code is removing some of the HTML elements.
You spans with classes citation and year are both inside your paragraph with class source. So the first time you reassign the innerHTML property of that paragraph, both of the spans are destroyed. Keep the spans and paragraph separate to resolve this issue.
Also, while this is not an error, you don't need to construct an anonymous function as a click event handler just to call another function that has no arguments. You can just establish that function as the click handler:
document.getElementById('loadQuote').addEventListener("click", printQuote);
Javier Gandia
Full Stack JavaScript Techdegree Student 9,153 PointsJavier Gandia
Full Stack JavaScript Techdegree Student 9,153 PointsSorry, if its a little difficult to read.. dont know how to write the code in here any other way