Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial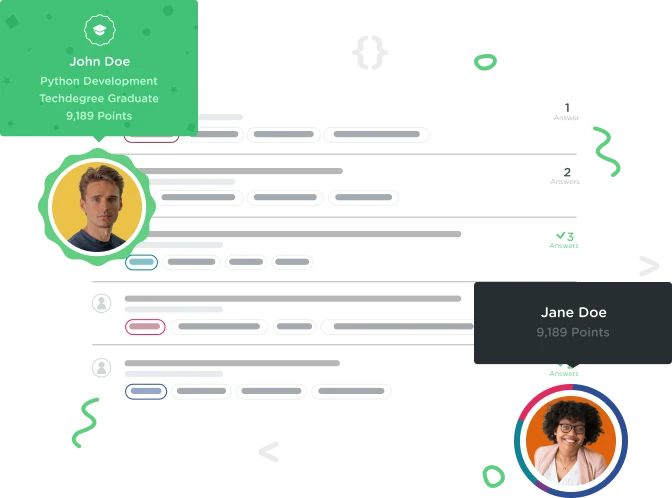

Zachary Martin
3,545 PointsWhy do we use a 2nd boolean here?
} public boolean applyGuess (char letter) { boolean isHit = mAnswer.indexOf(letter) >= 0; if (isHit) { mHits += letter; } else { mMisses += letter;
Why do we use the 2nd boolean here before isHit when we used it before applyGuess?
1 Answer
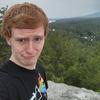
Brendon Butler
4,254 PointsSince
public boolean applyGuess() {
}
is a method, the "boolean" in this line is referring to the return type of the method.
This reference
boolean isHit = //blah blah blah
is a variable. In Java, variables aren't dynamic like in Python or other languages. So you have to tell the compiler what type of variable it is.
Say you have a light switch. This light switch has two methods -- a method to check if the light is on and a method to set the light on or off.
public class LightSwitch {
// this creates the variable and sets the default value
private boolean on = false;
// for this method you HAVE to return either true or false
public boolean isOn() {
return on; // returns the variable we created above
}
// for this method, it has the return type of void so you don't have to return anything.
public void turnOn() {
if (on) {
System.out.println("The light is already on!");
return; // you still can use the return statement to break out of the method without running any code below it
}
on = true;
}
public void turnOff() {
if (!on) {
System.out.println("The light is already off!");
return; // you still can use the return statement to break out of the method without running any code below it
}
on = false;
}
}