Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial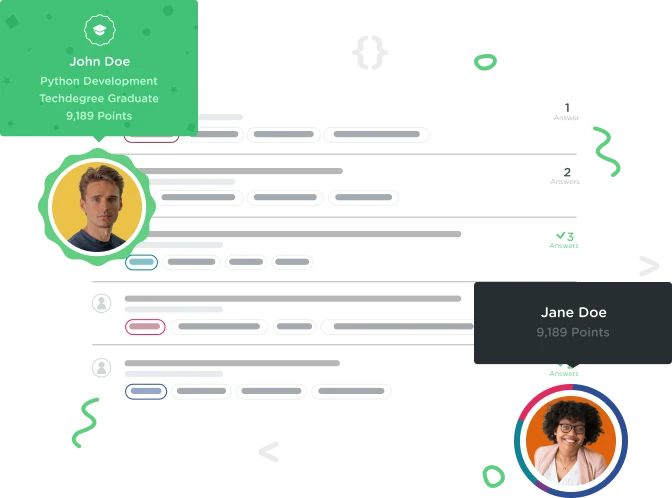

Abe Daniels
Courses Plus Student 2,781 PointsWhy doesn't this work?
I am completely blown away that I didn't get this one on the first try. It seems pretty straight forward. My return value of true/false is what seems to be the issue. I don't understand what other type of value it would be since i am working with a boolean.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
if (mHand) {
boolean hasTile = true;
return true;
} else {
return false;
}
}
public boolean hasTile(char tile) {
return false;
}
}
4 Answers

Chase Marchione
155,055 PointsHi Abe,
The issue is that mHand is a string variable, but your 'if (mHand)' test treats mHand as a boolean. That statement is interpreted as 'if mHand is true', which cannot be tested given that mHand is not a true/false data type, but a string.
Fortunately, you already have a statement that does what you need: updates mHand with the new tile. That statement is 'mHand += tile;'.
Shortening the code a little:
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return false;
}
}
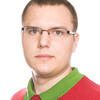
Maciej Torbus
8,137 PointsHey Abe!
Your method addTile is the type of void. Void methods should not return any value. I think that this method should look something like your first line only. It just adds tile to the hand.
On the other hand, the code you wrote is nearly correct, but you should move it to your hasTile method, which return boolean value
Try it on your own, good luck!

Abe Daniels
Courses Plus Student 2,781 PointsThe issue is that it's asking me to make an if else, inside of a boolean. So how would i incorporate that? I tried just sliding the code into the hasTile constructor but it didnt do the trick. I understand what the issue is, i just don't know how to make a solution. How do you go about translating a boolean, to work for a string object?

Cynthia Jenkins
4,983 PointsI'm fairly new at this (I did this one a couple days ago), but I believe your if statement needs a bit of work. Anyways, you were correct to put it all under hasTile, but you needed to adjust it to if (mHand.indexOf() > -1) {.... } else {}. Because it seems like you're saying .. well, if mHand ... then do this.. but if mHand is what? Otherwise I think what you had was correct.