Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial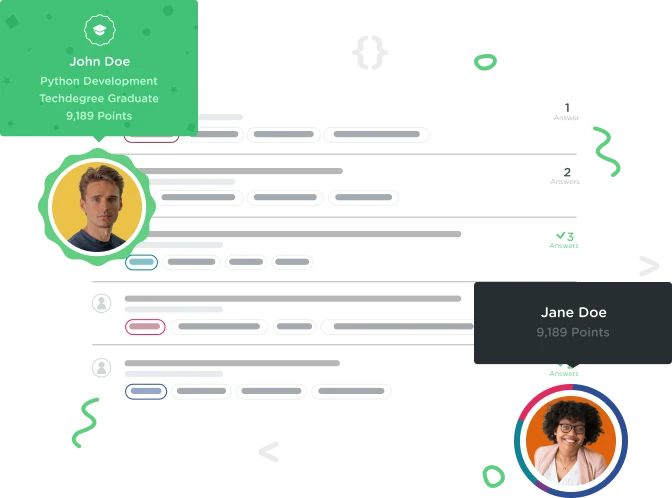
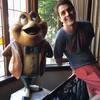
Kevin Jordan
3,953 PointsWhy is this code not being accepted?
I wrote this in VS 2017, it seems to run according to what is asked of the code, but isn't being accepted as an answer.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var yayNumber = Console.ReadLine();
var realNumber = 0;
var counter = 0;
try
{
while (true)
{
realNumber = int.Parse(yayNumber);
if (counter <= realNumber)
{
Console.WriteLine("Yay!");
counter += 1;
if (counter == realNumber)
{
break;
}
}
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
2 Answers
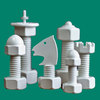
Steven Parker
241,809 PointsIt's apparently an infinite loop.
But first, I should mention that you only need to convert the input string to int
one time. So you can move it out of the loop and do it once before the loop starts.
Then, I'm guessing that for task 2 they test by entering a "0" value. The way you're controlling the loop iterations might work for positive numbers, but if 0 is entered it will get stuck running forever. But before you add code to check for that specifically let me show you another approach:
The condition clause of a while
is really intended to control when the loop stops. It might work to set it to true
and then force the loop to end using a break
, but it's much more concise to use it as intended. So you could shorten the parse and loop code to just this:
realNumber = int.Parse(yayNumber); // moved out of the loop
while (counter < realNumber)
{
Console.WriteLine("Yay!");
counter += 1;
}
Now you don't have to do it this way to pass the challenge (assuming you fix the 0 issue another way), but I feel better for showing it to you.
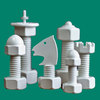
Steven Parker
241,809 Points
Be careful about testing a challenge in an external REPL or IDE.
If you have misunderstood the challenge, it's also very likely that you will misinterpret the results.
A program built according to the challenge instructions will prompt and take input one time only, and then either print some "Yay!" messages or an error message. Either way, it will then end.
Your program has an outer loop that causes it to repeat if an invalid number is entered.
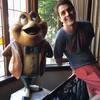
Kevin Jordan
3,953 PointsThanks for your response, I did remove the outer loop and try the code again in workspaces and it still failed the 2nd task. So I think it has something to do with the Catch area. I linked gifs below for proof.
1st Task: (Passed) https://gyazo.com/3d4cb51590e03cd54d92afaec668180e
2nd Task: (Failed) https://gyazo.com/71526f91657accae02badb63151dd2e2
I am digging into if it is a misunderstanding on my part, which is most likely right, but is hard to see due to cognitive bias.
Kevin Jordan
3,953 PointsKevin Jordan
3,953 PointsLike a true noob, I didn't check the "0". I did switch the try block to be outside and put the parse outside the while loop, this did stop the loop and shorten my code by a lot. I need to be more careful about using condition clauses as intended. Thank you for your help!