Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial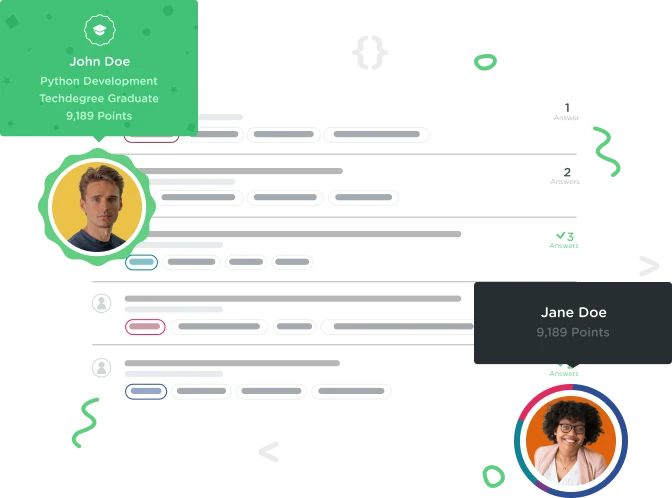
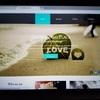
Kyle Jensen
Courses Plus Student 6,293 PointsWhy use Equals when == works just fine (override Equals())
Overriding of Equals() and then using it in the Path class as the teacher showed
public override bool Equals(object obj) {
if(!(obj is Point)) {
return false;
}
Point that = obj as Point;
return this.X == that.X && this.Y == that.Y;
}
public bool IsOnPath(MapLocation location) {
foreach(var pathLocation in _path) {
if (location.Equals(pathLocation)) {
return true;
}
}
return false;
}
}
is the same as not overriding it and doing this:
public bool IsOnPath(MapLocation location) {
foreach(var pathLocation in _path) {
if (location.X == pathLocation.X && location.Y == pathLocation.Y) {
return true;
}
}
return false;
}
}
either one is valid. I wondered if it was necessary to do this so I tested the second version to see if I could get the compiler to return true and it works just fine. I'm still unclear, at this time, why I'd waste the time writing the override AND the hash code just to get the same results from my test version.
Can someone please explain why I would ever bother to write all that extra code out if I don't have to??
1 Answer
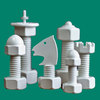
Steven Parker
231,124 PointsThese operations are not completely identical.
One performs a test for value equality and the other defaults to a test for reference equality. The functional difference varies with characteristics of the type the operators are applied to.
Further explanation can be found on this MSDN page that discusses how and when to override each of them.
But also, as your own example shows, using Equals can be a convenient shorthand for comparing individual properties. Plus it promotes encapsulation by keeping the knowledge of the internals of the class in the same module as its definition.